Table of Contents
ToggleIn this article we will into the ASCII table in C, exploring its various character sets—from control characters to printable characters, including letters, digits, and special symbols—and demonstrates how to interact with these characters through C programming.
What is ASCII Table In C?
The ASCII table is a character-encoding scheme originally based on the English alphabet. ASCII stands for American Standard Code for Information Interchange. It encodes 128 specified characters into seven-bit integers as shown by the ASCII standard. These characters include letters (both uppercase and lowercase), digits, punctuation marks, and non-printable control characters.
- ASCII control characters (0-31) are non-printable and used for text and device control, like carriage returns (ASCII 13).
- ASCII printable characters span from 32 to 126, including letters, numbers, and special symbols.
- Special characters are found in ranges 32-47, 58-64, 91-96, and 123-126, encompassing various punctuation marks and symbols.
- The numeric range 48-57 represents decimal digits 0-9.
- Alphabets are split into uppercase (65-90) and lowercase (97-122), covering all English alphabet letters.
ASCII Table
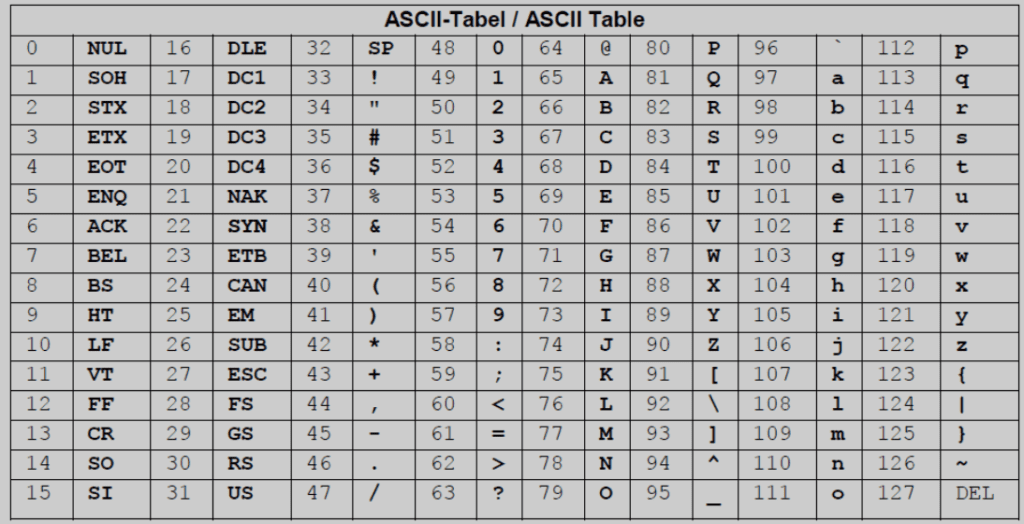
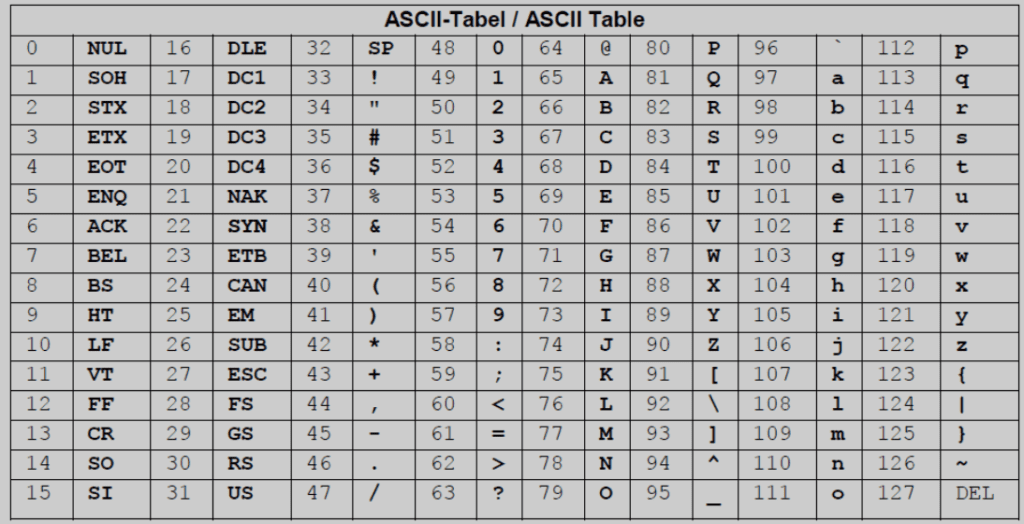
ASCII 8: Extended ASCII Characters
The Extended ASCII is an 8-bit character set that includes the standard ASCII characters (0-127) and an additional 128 characters (128-255), bringing the total to 256. These additional characters include various symbols, diacritics, and graphical symbols. However, it’s important to note that the extended ASCII can vary depending on the system or standard being used (such as ISO-8859-1 or Windows-1252).
Programs To Print ASCII Value in C
Let’s look some of the C programs to print ascii value.
C Program to Print ASCII Value of 0 to 9
#include <stdio.h>
int main() {
for(char c = '0'; c <= '9'; c++) {
printf("ASCII value of %c is %d\n", c, (int)c);
}
return 0;
}
Output
ASCII value of 0 is 48
ASCII value of 1 is 49
ASCII value of 2 is 50
ASCII value of 3 is 51
ASCII value of 4 is 52
ASCII value of 5 is 53
ASCII value of 6 is 54
ASCII value of 7 is 55
ASCII value of 8 is 56
ASCII value of 9 is 57
Explanation: This program iterates from the character ‘0’ to ‘9’, converting each character to its ASCII integer value using type casting (int(c)) and prints it.
C Program To Print ASCII Value of a Character
#include <stdio.h>
int main() {
char c;
printf("Enter a character: ");
scanf("%c", &c);
printf("ASCII value of %c is %d\n", c, (int)c);
return 0;
}
Output:
ASCII value of A is 65
Explanation: This program prompts the user to enter a character, then prints the ASCII value of the entered character by casting the character to an integer.
C Program to Print ASCII Value of A to Z using Type Conversion
#include <stdio.h>
int main() {
for(char c = 'A'; c <= 'Z'; c++) {
printf("ASCII value of %c is %d\n", c, (int)c);
}
return 0;
}
ASCII value of A is 65
ASCII value of B is 66
ASCII value of C is 67
...
ASCII value of X is 88
ASCII value of Y is 89
ASCII value of Z is 90
Explanation: In the above code, printf
is used for output, and the character c
is cast to an integer (int)c
to display its ASCII value, which follows C’s standard for type conversion and input/output operations.
Why is ASCII Table In C Important?
The ASCII table is crucial in C programming because it provides a standard way to represent characters as integer values, facilitating text manipulation and communication between systems. It enables programmers to perform operations on characters, such as comparisons, conversions, and calculations, with ease. Additionally, understanding ASCII is essential for handling data input/output, encoding, and processing in various programming scenarios, making it fundamental for effective coding and system interoperability.
Check out
More C tutorials here
I hope You liked the post 👍. For more such posts, 📫 subscribe to our newsletter. Try out our free resume checker service where our Industry Experts will help you by providing resume score based on the key criteria that recruiters and hiring managers are looking for.
FAQ
What is ASCII 7 and ASCII 8?
ASCII 7, also known as 7-bit ASCII, is a character encoding standard that uses 7 bits to represent 128 unique characters, including letters, digits, and control characters. ASCII 8, or Extended ASCII, expands on this by using an extra bit (making it 8 bits in total) to represent 256 characters, allowing for additional symbols, special characters, and international characters not found in the standard ASCII set.
How to write ASCII code?
Writing ASCII code involves using numeric values to represent characters in text. For example, in programming, you can use the numeric ASCII value with a specific syntax (like \x41
for ‘A’ in C++ or Python) to insert characters into strings or to perform character operations. This approach allows programmers to directly manipulate text at its fundamental, numeric level, enabling precise control over data processing and display tasks.