Table of Contents
ToggleIn this article we will look into basic structure of C program. we will understand the six fundamental building blocks that comprise a complete C program, providing examples and explanations of each component. Whether you are learning C for the first time or looking to strengthen your foundational knowledge, this article will provide an excellent introduction to writing properly structured C code.
So, let’s get started.
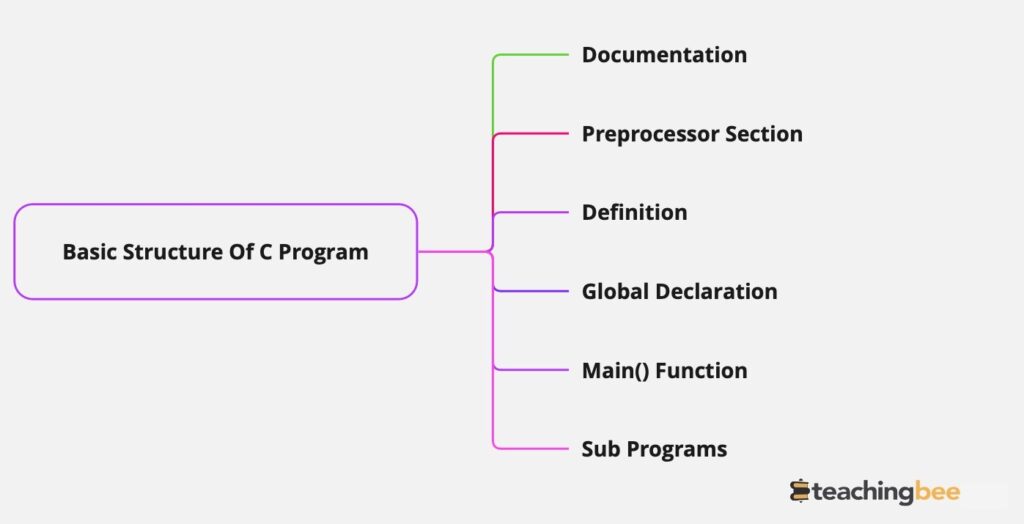
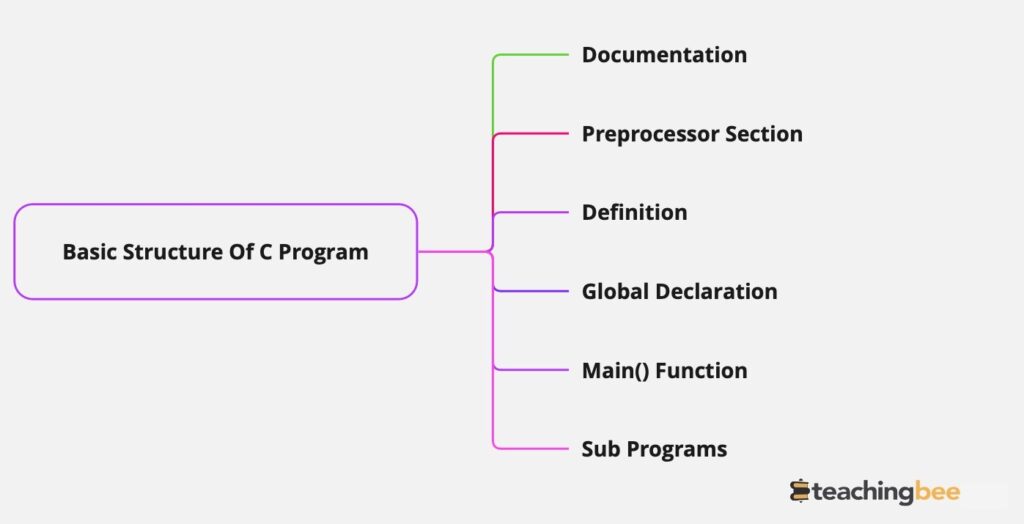
The basic structure of C program consists of 6 sections these are:
- Documentation
- Preprocessor Section
- Definition
- Global Declaration
- Main() Function
- Sub Programs
Let’s understand each of these section in detail using examples:
Basic Structure Of C Program
The structure of C program is organised into distinct sections, each serving a specific purpose, including documentation, preprocessor directives, definitions, global declarations, the main function, and subprograms, contributing to a well-organised and executable codebase.Let’s understand each in detail.
Documentation Section
Documentation section in C program at the beginning of the program provides details about what the program does, who wrote it, version info etc. It makes it easier for someone else reading your code to understand your program.
/*
Program: Add two numbers
Author: John Doe
Date: 01/01/2023
Description: This program takes two integer inputs from the user and prints their sum
*/
The documentation section is not a formal section in C, but it’s good practice to include comments at the beginning of your program to provide information about the program, its purpose, the author, creation date, and any other relevant details.
Good documentation should have:
- Purpose of program
- Author details (name, contact info etc.)
- Date created/updated
- Version info
- Details on modules/functions
- Input and output details
- Any assumptions or dependencies
Preprocessor Section
Preprocessor section has preprocessor directives that tell the compiler to include header files. Header files allow you to access predefined functions.This section starts with the #
symbol and includes preprocessor directives. These directives are processed before the actual compilation of the code.
#include <stdio.h>
Commonly used preprocessor directives:
Directive | Purpose |
---|---|
#include | Includes header files containing definitions of functions and variables to be used in the program. |
#pragma | Issues special commands directly to the compiler to control compilation. Allows enabling/disabling warnings etc. |
#undef | Undefines an existing #define macro. Allows selectively undefining macros that were defined earlier. |
#if, #ifdef, #ifndef | Allows conditional compilation. Code blocks can be compiled selectively based on conditional expressions involving defined macros. Useful for debugging. |
Definition Section
This section defines symbolic constants using #define. It is used to assign meaningful names to values that enhance readability.
#define MAX_SIZE 100
Global Declaration
The global declaration section contains declarations of global variables and functions that can be accessed from anywhere in the program.
int sum; //global variable
void add(); //function prototype
Main() function
This is the most important section and contains the main logic of the program. The main
function is the entry point of a C program. It is mandatory for every C program and is where the program begins its execution.
int main() {
// code
return 0;
}
Sub Programs / User-defined functions
Sub programs refer to functions other than main
that you define in your program. These functions can be called from main
or other functions.This section contains additional functions defined by the programmer for modular programming.
// Function definition
void myFunction() {
// Code for the function
}
int main() {
myFunction(); // Calling the function
return 0;
}
Putting it all together, a simple C program might look like this:
/* Section 1: Documentation */
/* This is a simple C program that demonstrates the basic structure */
/* Author: John Doe */
/* Date: January 1, 2023 */
/* Section 2: Preprocessor Section */
#include <stdio.h>
/* Section 3: Definition */
#define PI 3.14159
#define MAX_SIZE 100
/* Section 4: Global Declaration */
int globalVariable; // Declaration of a global variable
void myFunction(); // Function declaration
/* Section 5: Main() Function */
int main() {
// Code goes here
myFunction(); // Calling the function
return 0;
}
/* Section 6: Sub Programs */
// Function definition
void myFunction() {
// Code for the function
}
Compilation and execution of a C program
For Windows Using MinGW
- Write your C program and save it (e.g., “myprogram.c”).
- Open Command Prompt and Navigate to Program Directory:
cd path\to\your\program
- If you don’t have MinGW installed, download and install it. Ensure that the MinGW/bin directory is added to your system’s PATH.
- Compile C Program using following command
gcc -o outputFileName yourProgramFileName.c
- Run Compiled Program. If your compiled program is named, for instance, “outputFileName.exe,” you would run:
outputFileName.exe
- Check the Command Prompt for program output.
- If needed, debug errors, modify the C code, and repeat compilation and execution.
Adjust paths and file names as necessary based on your specific program and directory structure.
For Linux
Checkout detailed steps on how to compile C program in Ubuntu here
Key Takeaways
- A C program generally consists of six sections: documentation, the preprocessor directives, definitions, global declarations, the main() function, and user-defined sub programs/functions.
- The documentation provides high-level details about the program to aid future maintenance and updates.
- The preprocessor directives allow you to include external libraries and set compilation conditions.
- Definitions establish symbolic constants for better readability, while global declarations enable the sharing of variables and functions across code.
- The main() function houses the key program logic and serves as the initial entry point when execution begins. Sub programs compartmentalize code into reusable modules with discrete tasks.
Checkout more C Tutorials here.
I hope You liked the post 👍. For more such posts, 📫 subscribe to our newsletter. Try out our free resume checker service where our Industry Experts will help you by providing resume score based on the key criteria that recruiters and hiring managers are looking for.
FAQ
What is the significance of the main()
function in a C program?
The main()
function is the entry point of a C program. When a C program is executed, it starts executing from the main()
function. It is mandatory for every C program, and any code you want to be executed must be placed within or called from the main()
function.
What is the purpose of the preprocessor directives #define
and #include
in C?
The #define
directive is used to define macros or symbolic constants, providing a way to create short names for values or code snippets. On the other hand, the #include
directive is used to include the content of header files in the source code. This is crucial for using functions and declarations from external libraries or modules.
Why is the order of sections (Documentation, Preprocessor, Definition, etc.) important in a C program?
The order of sections in a C program is important because the C compiler processes the program sequentially. For example, if a function is used before it is declared, the compiler may generate an error. Similarly, preprocessor directives like #define
should be placed before they are used. Proper section ordering ensures that the program is logically structured and can be compiled without errors.