Table of Contents
ToggleIn programming, we often follow a straight path, executing one statement after another. However, there are times when we need to make choices and decide which parts of our code should run based on certain conditions. This is where conditional processing comes into play, acting like a crossroads in our code, guiding the execution flow in different directions.
In C programming, these statements are fundamental for creating dynamic and efficient programs. This blog post delves into the various types of decision control statements in C, providing a comprehensive guide for beginners and experienced programmers.
What Are Decision Control Statements?
Decision control statements in C dictate the execution flow based on certain conditions. These statements evaluate conditions and, depending on whether the conditions are true or false, execute the corresponding code block. They are essential for implementing logic in your programs, making them behave differently under different conditions.
Types of Decision Control Statements in C
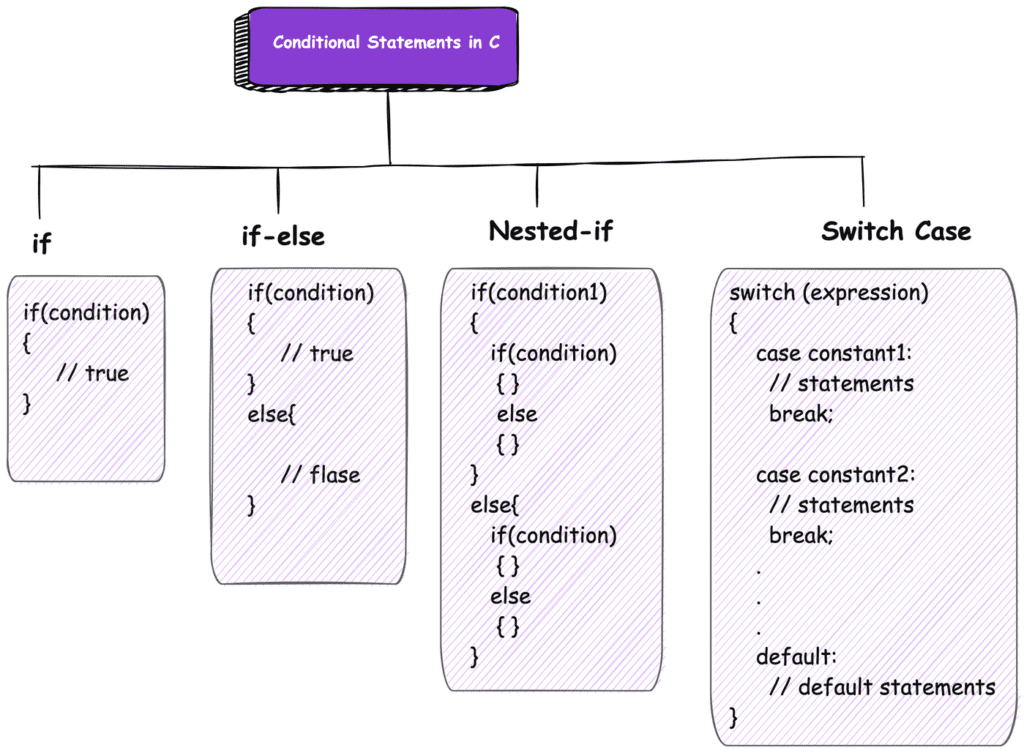
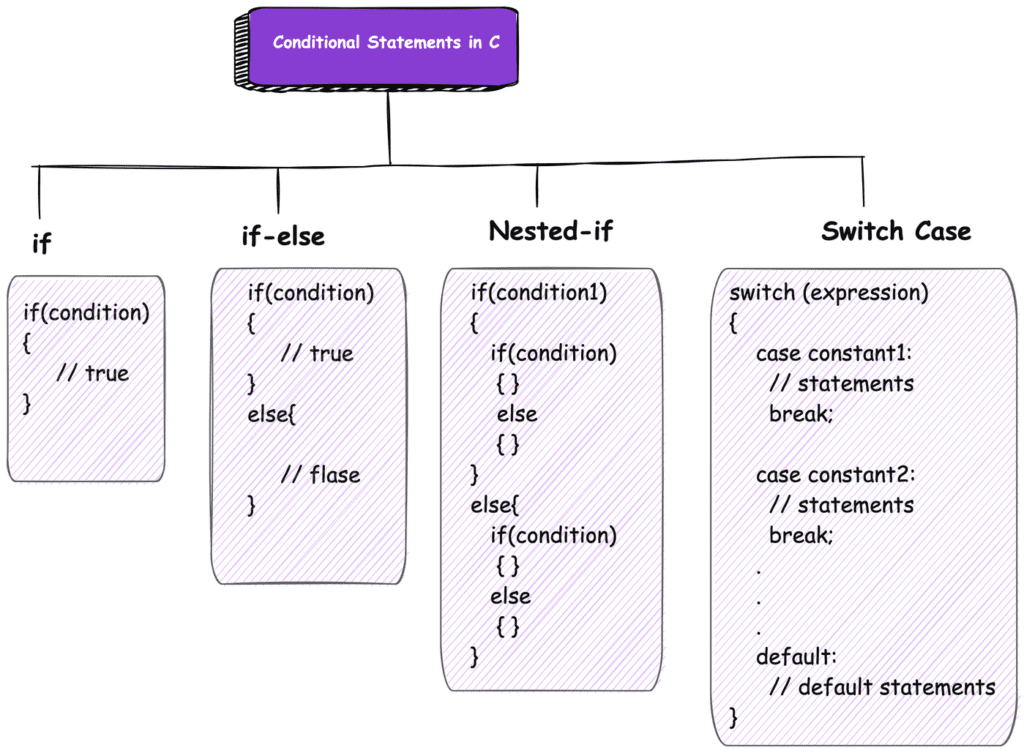
There are several types of decision control statements in C, each serving a unique purpose:
Simple if Statement
The if
statement evaluates a Boolean condition inside parentheses and executes the code block if the condition is true.
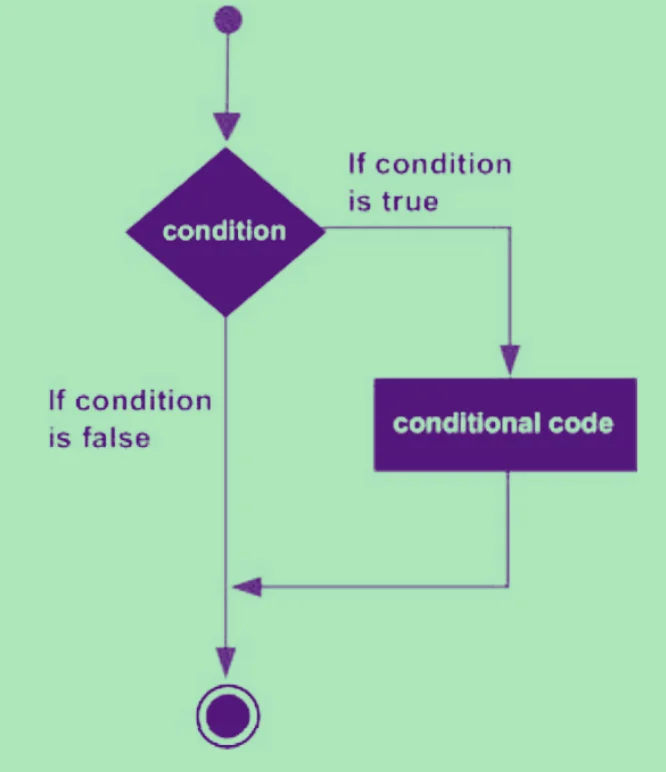
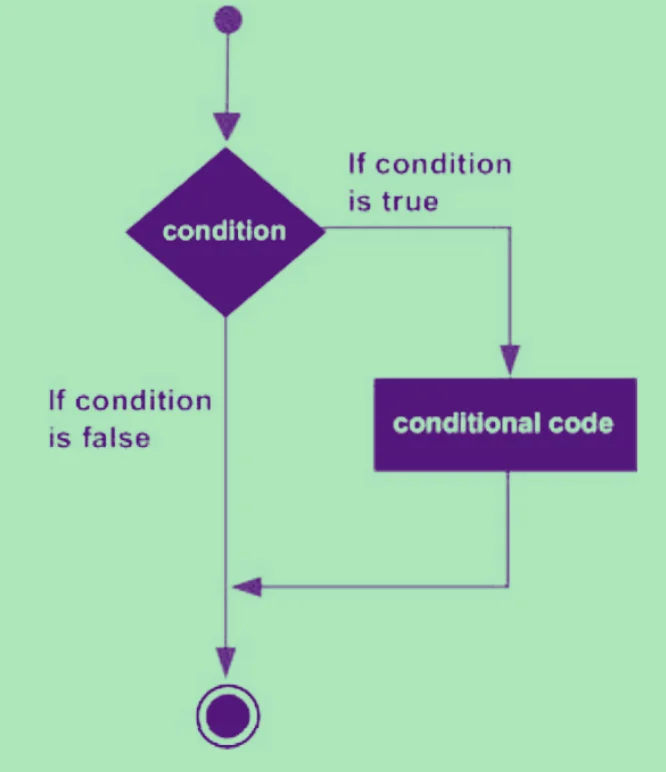
Syntax: if (condition)
{
// code to execute if condition is true
}
Example: Checking if a number is positive.
#include <stdio.h>
int main() {
int number = 5;
if (number > 0) {
printf("The number is positive.\n");
}
return 0;
}
Output:
The number is positive.
This program checks if a number is positive. If the number is greater than 0, it prints “The number is positive.” In this case, since the number is 5, the program will print the message.
If-else Statement
The if-else statement extends the functionality of a simple if statement by allowing two separate code blocks – one that runs when the condition is true, and one that runs when it is false.
Syntax: if (condition) {
// code if true
}
else {
// code if false
}
Example: Determining if a number is even or odd.
#include <stdio.h>
int main() {
int number = 4;
if (number % 2 == 0) {
printf("The number is even.\n");
} else {
printf("The number is odd.\n");
}
return 0;
}
Output:
The number is even.
This program determines if a number is even or odd. If the remainder when the number is divided by 2 is 0, it prints “The number is even.” Otherwise, it prints “The number is odd.” Since 4 is even, the output will be “The number is even.”
Nested if-else Statements
Nested if-else statements involve if-else blocks within other if-else blocks. This allows evaluating multiple conditionals and branching to different code sections based on the outcomes.
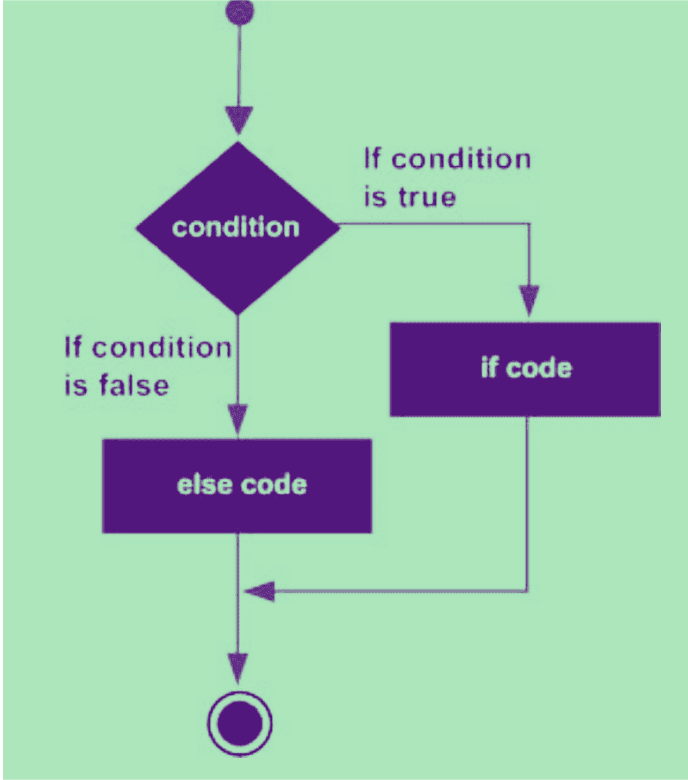
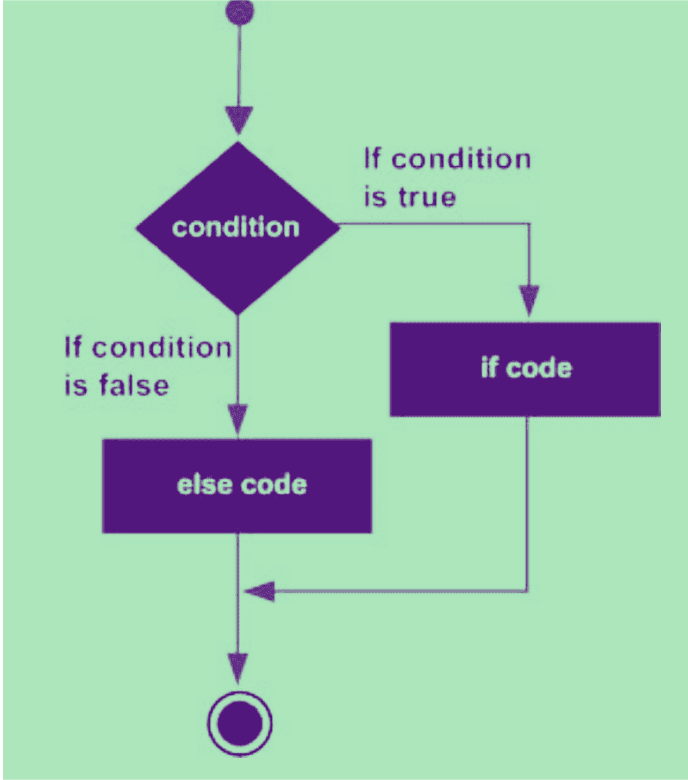
Syntax: if (condition)
if (x > 10) {
if (y > 5) {
// code A
}
else {
// code B
}
}
else {
if (z > 2) {
// code C
}
else {
// code D
}
}
Example: Finding the largest of three numbers.
#include <stdio.h>
int main() {
int a = 10, b = 20, c = 15;
if (a > b) {
if (a > c) {
printf("a is the largest number.\n");
} else {
printf("c is the largest number.\n");
}
} else {
if (b > c) {
printf("b is the largest number.\n");
} else {
printf("c is the largest number.\n");
}
}
return 0;
}
Output:
b is the largest number.
This program finds the largest of three numbers using nested if-else statements. It first compares a
and b
, and within each branch, it compares the larger of a
and b
with c
. In this case, b
is the largest number, so it prints “b is the largest number.”
Switch Case Statements
The switch case statement allows selection of code blocks to execute based on the value of an expression.The switch case operates by evaluating an expression, typically an integer or a character, and then jumping to the corresponding case label that matches the evaluated result. If none of the case labels match, the program executes the default block if it is provided.
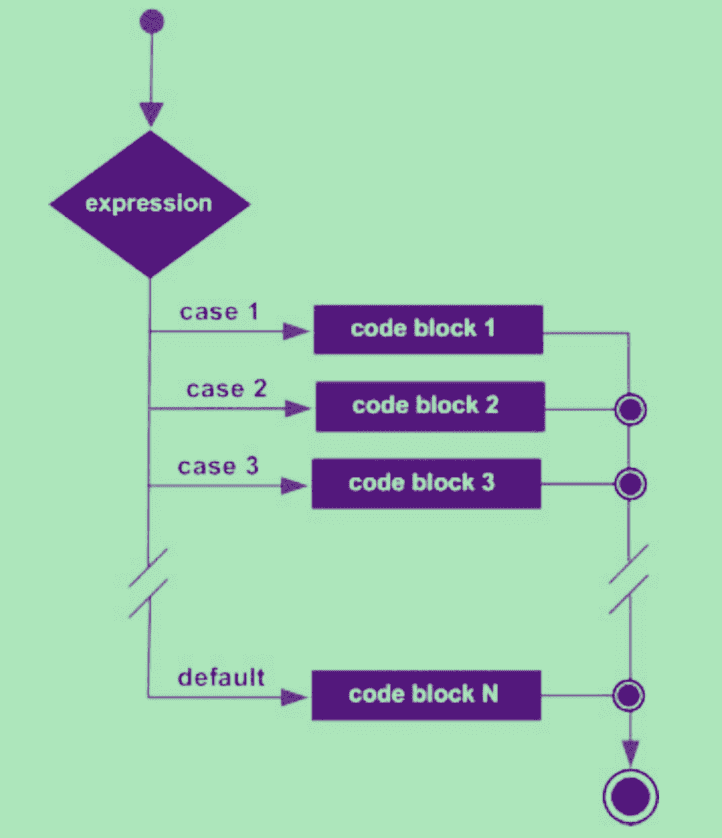
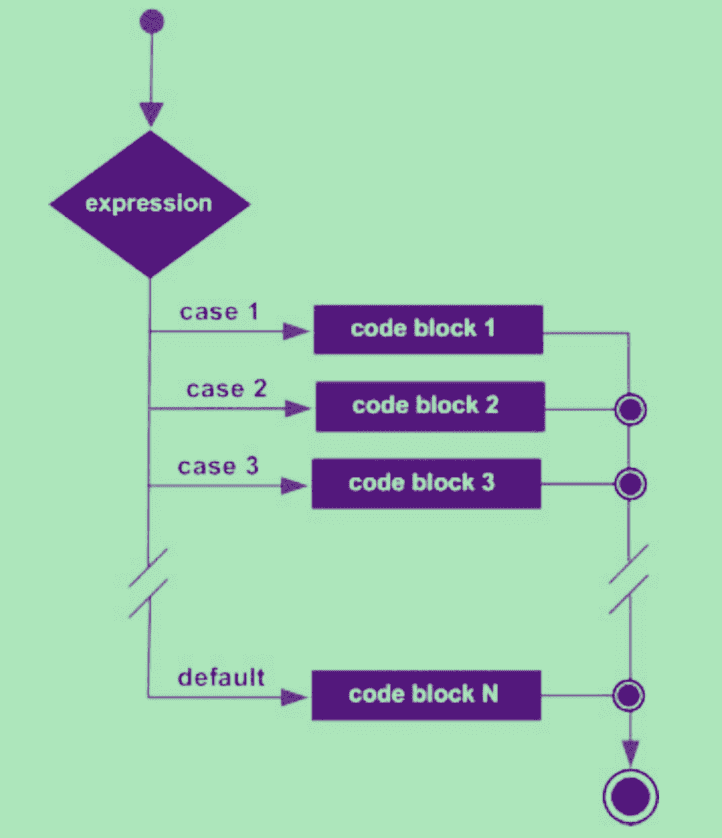
The syntax of a switch case statement looks like this:
Syntax:
switch(expression) {
case constant1:
// code to be executed if expression == constant1;
break;
case constant2:
// code to be executed if expression == constant2;
break;
// ... more cases ...
default:
// code to be executed if expression doesn't match any case;
}
Example:
#include <stdio.h>
int main() {
int day = 4;
switch(day) {
case 1:
printf("Monday\n");
break;
case 2:
printf("Tuesday\n");
break;
case 3:
printf("Wednesday\n");
break;
case 4:
printf("Thursday\n");
break;
case 5:
printf("Friday\n");
break;
case 6:
printf("Saturday\n");
break;
case 7:
printf("Sunday\n");
break;
default:
printf("Invalid day\n");
}
return 0;
}
Output:
Thursday.
This program takes a numerical day of the week and prints the name of the day using a switch case statement. The day
variable is used as the expression in the switch, and each case corresponds to a day of the week. Since day
is 4, the program prints “Thursday.” Here we have hard coded the variable value but instead we can take input from user as well.
Why Use Switch Case?
- Clarity: The switch case provides a clear and concise way to handle multiple conditions, making the code easier to read and understand.
- Efficiency: It is often more efficient than multiple if-else statements, especially when dealing with many conditions.
- Maintainability: Adding or removing cases is now easier with less chance of errors, leading to more maintainable code.
Best Practices
- Always Include a Break: Don’t forget to include a
break
statement at the end of each case (unless you have a specific reason to use fall-through behavior). This prevents the execution from falling through to the next case. - Use Default Wisely: Include a
default
case to handle unexpected values. Even if you think every possible value is covered by a case, having a default ensures that your program can handle surprises gracefully. - Keep Cases Simple: Each case should be simple and quick to execute. If complex logic is needed, consider calling a function from the case instead of writing all the logic inside the case.
Wrapping Up
In this blog, we’ve talked a lot about Decision Control Statements in C programming. We looked at different ways to do this, like using if statements, if-else, and switch cases. These are like crossroads in our code, helping us decide which way to go based on what’s happening in our program. We used simple examples to make sure everything is clear and easy to understand.
Checkout more C Tutorials here.
I hope You liked the post ?. For more such posts, ? subscribe to our newsletter. Try out our free resume checker service where our Industry Experts will help you by providing resume score based on the key criteria that recruiters and hiring managers are looking for.