Table of Contents
ToggleWhat is the perfect number in C?
A perfect number in C, is a positive integer that is equal to the sum of its proper divisors, excluding the number itself. This means that if you add up all the numbers that divide perfectly into a given number (without leaving a remainder) and exclude the number itself from this sum, the total will equal the original number if it’s perfect.
For example, 6 is a perfect number. Its divisors are 1, 2, and 3. If you add these divisors together (1 + 2 + 3), the sum is 6, which is the original number. Hence, 6 is considered a perfect number.
Algorithm to Find Perfect Number
- Initialize Sum: Start with a sum of 0. This will be used to accumulate the sum of the divisors.
- Find Divisors: Loop through numbers from 1 up to (but not including) the given number.
- Check Divisibility: For each number in the loop, check if it is a divisor of the given number (i.e., the given number divided by this number leaves no remainder).
- Accumulate Divisors: If a number is found to be a divisor, add it to the sum.
- Check for Perfection: After all divisors have been considered, compare the sum of divisors to the original number. If they are equal, the number is perfect.
Pseudocode:
IsPerfectNumber(n)
Begin
sum ← 0
// Loop from 1 to n-1
For i ← 1 to n-1 do
// Check if i is a divisor of n
If n mod i = 0 then
sum ← sum + i
EndIf
EndFor
// Check if sum of divisors equals the number
If sum = n then
Return True
Else
Return False
EndIf
End
Program to Check Perfect Number in C
There are three main ways to check for perfect number in C:
- Using for Loop
- Using while Loop
- Using recursion
Using for Loop
Program to check whether the number taken from a user is perfect or not in C
#include <stdio.h>
int sumOfDivisors(int num) {
int sum = 0; // Initialize sum of divisors
// Loop to find and add up the divisors of num
for (int i = 1; i <= num / 2; i++) {
if (num % i == 0) {
sum += i; // Add the divisor to the sum
}
}
return sum;
}
int main() {
int num, sum;
printf("Enter a number: ");
scanf("%d", &num);
sum = sumOfDivisors(num);
// Check if the sum of divisors is equal to the number
if (sum == num) {
printf("%d is a perfect number.\n", num);
} else {
printf("%d is not a perfect number.\n", num);
}
return 0;
}


Using while Loop
In this method, we will use a while loop to check whether the given number is a perfect number or not.
#include <stdio.h>
int sumOfDivisors(int num) {
int sum = 0; // Initialize sum of divisors
int i=1;
// Loop to find and add up the divisors of num
while (i <= num / 2) {
if (num % i == 0) {
sum += i; // Add the divisor to the sum
}
i++;
}
return sum;
}
int main() {
int num, sum;
printf("Enter a number: ");
scanf("%d", &num);
sum = sumOfDivisors(num);
// Check if the sum of divisors is equal to the number
if (sum == num) {
printf("%d is a perfect number.\n", num);
} else {
printf("%d is not a perfect number.\n", num);
}
return 0;
}
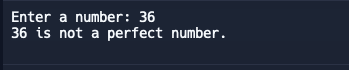
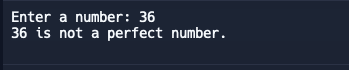
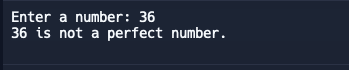
Using Recursion
To check if a number is a perfect number using recursion in C, we can write a recursive function that sums the divisors of the number.
#include <stdio.h>
// Recursive function to find the sum of divisors of 'num'
// 'i' is the current divisor to check
int sumOfDivisorsRecursively(int num, int i) {
// Base case: if 'i' is equal to the number, return 0
// No need to go beyond num / 2, as no divisors exist beyond num / 2 for any number
if (i == num / 2 + 1) return 0;
// Check if 'i' is a divisor of 'num', if so add 'i' to the sum
// Recursively call the function with 'i' incremented by 1
return ((num % i == 0) ? i : 0) + sumOfDivisorsRecursively(num, i + 1);
}
// Function to check if a number is perfect using recursion
void checkPerfectNumber(int num) {
// Initial call to the recursive function with 'i' starting from 1
int sum = sumOfDivisorsRecursively(num, 1);
// If the sum of divisors is equal to the number, it's a perfect number
if (sum == num)
printf("%d is a perfect number.\n", num);
else
printf("%d is not a perfect number.\n", num);
}
int main() {
int num;
printf("Enter a number: ");
scanf("%d", &num);
checkPerfectNumber(num);
return 0;
}
- The
sumOfDivisorsRecursively
function takes two parameters:num
(the number to check) andi
(the current divisor being checked). It starts withi = 1
. - In the base case, when
i
reachesnum / 2 + 1
, the function returns 0, as no divisors can be greater thannum / 2
. - For each recursive call, the function checks if
i
is a divisor ofnum
. If it is,i
is added to the sum. Regardless, the function calls itself withi + 1
. - The
checkPerfectNumber
function initiates the recursive process and checks if the sum returned bysumOfDivisorsRecursively
equalsnum
. It then prints the result accordingly. - In
main
, the program prompts the user for a number, which is then passed tocheckPerfectNumber
to determine if it’s a perfect number.
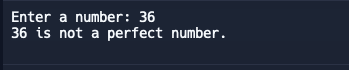
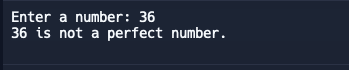
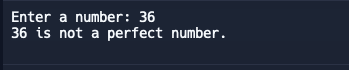
Perfect Numbers between given range
What are the perfect numbers from 1 to 100?
There are only two perfect numbers from 1 to 100 and those are 6 and 28.
C Program to Find Perfect Number between 1 to 100
Below is a C Program to Find Perfect Number between 1 to 100. The idea is to iterate through numbers from 1 to 100 and check if each number is a perfect number or not. The program utilises a function to sum the divisors of each number and then compares this sum to the number itself to check if the number is perfect.
#include <stdio.h>
// Function to calculate the sum of divisors
int sumOfDivisors(int num) {
int sum = 0;
for (int i = 1; i <= num / 2; i++) {
if (num % i == 0) {
sum += i; // Add divisor to sum
}
}
return sum;
}
// Main function to find and print perfect numbers between 1 and 100
int main() {
printf("Perfect numbers between 1 and 100 are: ");
for (int num = 1; num <= 100; num++) {
if (num == sumOfDivisors(num)) {
printf("%d ", num);
}
}
printf("\n");
return 0;
}
Output


Explanation
- The
sumOfDivisors
function calculates the sum of all proper divisors of a given numbernum
. It iterates from 1 tonum / 2
because no divisor of a number can be greater than half of that number (except the number itself, which isn’t a proper divisor). - The
main
function iterates through numbers from 1 to 100, using thesumOfDivisors
function to find the sum of each number’s divisors. If a number is equal to the sum of its divisors, it’s printed out as a perfect number.
Conclusion
In this blog post, we talked about perfect number in C. Perfect numbers are special because they are equal to the sum of their smaller parts. We showed different ways to do this, like using loops and a method called recursion. Understanding how to find perfect numbers in C is a good way to get better at programming and learn more about how numbers work.
For more C related blogs click here.