Table of Contents
ToggleIn this article, we will explore the fundamental aspects of strings in C, including declaration, initialization, and various operations such as input, length calculation, and manipulation using string.h
library functions. We’ll also delve into the differences between character arrays and string literals, and discuss strings in the context of pointers. Along the way, we’ll include numerous code snippets to illustrate key points.
What Is String In C Language?
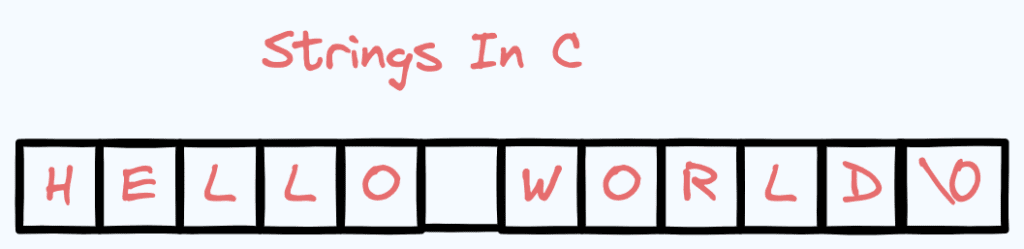
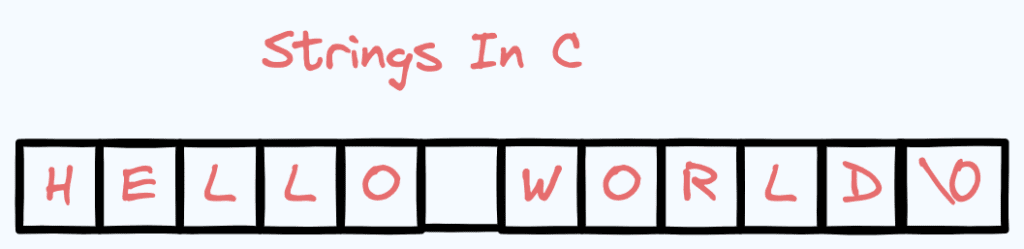
In C, a string is a sequence of characters terminated by a null character '\0'
. This null character signifies the end of the string and is essential for various string manipulation operations. Strings are stored in arrays of char
type, making them closely related to character arrays.
String Syntax In C : String Declaration
Declaring a string in C can be done by specifying an array of characters. The syntax for declaration is as follows:
char str[size];
Where size
is the number of characters the string can hold, including the null terminator. It’s important to ensure the array is large enough to store all characters of the string plus the null terminator.
String Syntax In C : String Initialization
Strings can be initialized in several ways at the time of declaration. Here are a few examples:
Assigning a String Literal without Size
char str[] = "Hello, World!";
In this case, the compiler automatically calculates the size of the array to fit the string literal plus the null terminator.
Assigning a String Literal with a Predefined Size
char str[50] = "Hello, World!";
Here, the array size is explicitly specified. If the string is shorter than the array, the rest of the array remains uninitialized.
Assigning Character by Character with Size
char str[14] = {'H', 'e', 'l', 'l', 'o', ',', ' ', 'W', 'o', 'r', 'l', 'd', '!', '\0'};
This method explicitly lists all characters, including the null terminator, with a predefined size.
Assigning Character by Character without Size
In C programming, strings can be assigned character by character without pre-declaring a fixed size. The characters are inserted into a character array one by one. A null terminating character ‘\0’ is added at the end to mark the end of the string. The compiler automatically determines the size of the array based on the number of characters assigned to it.
char str[] = {'H','e','l','l','o',' ','W','o','r','l','d','!','\0'};
It’s not recommended to assign characters one by one without specifying the size because it can lead to errors if the null terminator is omitted accidentally. Always ensure the size is accounted for or use string literals for initialization.
Reading and Writing Strings In C
Reading and writing strings is essential for taking user input and displaying output in C programming. To read string input, the scanf() and fgets() functions are used, with %s format specifier for scanf(). For printing output strings, printf() and puts() functions are available, again using %s in printf() to print the string contents.Let’s look each of these in detail.
How To Read String In C?
To read a string from the user in C, we can use scanf() function along with %s format specifier.
#include <stdio.h>
int main() {
char name[50];
printf("Enter your name: ");
scanf("%s", name);
printf("Your name is: %s", name);
return 0;
}
The %s format specifier allows scanf() to read a string input from the user and store it into the char array/string – name.
We can also use fgets() function to read an entire line including spaces as input string.
fgets(name, 50, stdin); // reads string with spaces
How To Print String In C?
To print a string in C, we can simply use printf() function with %s format specifier.
#include <stdio.h>
int main() {
char str[50] = "Hello World";
printf("%s", str);
return 0;
}
The string inside double quotes is stored into char array str, and %s prints the entire content of this string character by character.
We can also print strings using puts() function:
puts(str); // prints string and newline
So in summary, scanf()/fgets() is used to read string input and printf()/puts() is used to print strings in C.
How To Read a String By Taking Input from the User?
To read a string from user input, scanf
and gets
functions can be used, but gets
is unsafe and deprecated due to the risk of buffer overflow.
Here’s a safe way using scanf
:
char str[100];
printf("Enter a string: ");
scanf("%99s", str); // Reads a string until a whitespace is encountered
Using gets:
char name[50];
// Using gets (unsafe, causes buffer overflow)
printf("Enter name again: ");
gets(name);
printf("Name: %s\n", name);
How to Read a String Separated by Whitespaces in C?
To read a string that includes whitespaces, fgets
is a safer alternative:
char str[100];
printf("Enter a string: ");
fgets(str, sizeof(str), stdin); // Reads until a newline or EOF is encountered
String Operations In C
Let’s look into some of the important string operations in C.
How to find length in string in C?
The strlen
function from the string.h
library is used to find the length of a string (excluding the null terminator):
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
printf("Length: %lu\n", strlen(str));
return 0;
}
// Output: Length: 13
Passing Strings to Functions
Strings can be passed to functions by simply passing the array:
void printString(char str[]) {
printf("%s\n", str);
}
Converting String to Number In C
Converting a string to a number is a common task in programming. In C, there are a few ways to perform this conversion:
Using atoi() function
The atoi() function takes a string as an argument and returns its value converted to an integer.
#include <stdio.h>
#include <stdlib.h>
int main() {
char str[10] = "12345";
int x = atoi(str);
printf("String value = %s\n", str);
printf("Integer value = %d", x);
return 0;
}
Using strtol() function
The strtol() function converts a string to a long integer. An advantage over atoi() is that it can handle valid numbers that overflow the int type.
long y = strtol(str, NULL, 10);
Manually parsing the string
We can also manually parse the string and accumulate the integer value character by character:
int value = 0;
for(int i = 0; str[i] != '\0'; i++) {
value = value*10 + (str[i] - '0');
}
This loops through each character, converts it to its integer equivalent by subtracting ASCII value of ‘0’, and keeps accumulating the number.
Strings and Pointers in C
Strings can also be manipulated using pointers. For instance, a string can be declared as a pointer to a character:
char *str = "Hello, World!";
This declaration makes str
a pointer to the first character of the string literal.
List of Standard C Library – String.h Functions
The string.h
library provides numerous functions for string manipulation, including:
Function | Description |
---|---|
strcpy(s1, s2) | Copies string s2 into string s1 |
strcat(s1, s2) | Concatenates string s2 onto the end of string s1 |
strlen(s1) | Returns the length of string s1 |
strcmp(s1, s2) | Compares string s1 and s2 |
strchr(s, ch) | Returns a pointer to the first occurrence of character ch in string s |
strstr(s1, s2) | Returns a pointer to the first occurrence of string s2 in string s1 |
memset(s, ch, n) | Fills the first n characters of string s with character ch |
memcpy(dest, src, n) | Copies n characters from string src to dest |
memmove(dest, src, n) | Same as memcpy, but handles overlapping strings |
strtok(s1, del) | Breaks string s1 into tokens separated by delimiters del |
atoi(s) | Converts string s to integer |
itoa(n, s) | Converts integer n to string representation in s |
Difference Between Character Array and String Literal
Here is the difference between character array and string literal in C provided in a tabular format:
Basis | Character Array | String Literal |
---|---|---|
Definition | Array of characters terminated by ‘\0’ | Sequence of characters enclosed within double quotes |
Memory | Occupies memory according to size declared | Occupies memory to accommodate characters in string plus ‘\0’ |
Modification | Can be modified | Cannot be modified (read-only memory) |
Usage | Used when string size may change | Used for fixed size strings |
Initialization | Must be initialized explicitly | Automatically initialized |
Example | char str[100]; | “hello world” |
Array Operations | Array operations like indexing can be performed | Array operations cannot be performed |
sizeof operator | Yields size of array | Yields size of string minus terminating NULL char |
Key TakeAways
- Strings in C are arrays of characters terminated by a null character ‘\0’. They are stored in char arrays and can be manipulated using various string functions.
- Strings can be declared by specifying a char array of fixed size, without size (allowing automatic size calculation), or assigned character by character. Initialization can be done using string literals or individual character assignments.
- The difference between character arrays and string literals is that character arrays are modifiable, occupy memory dynamically, and need explicit initialization, while string literals are stored in read-only memory, have fixed sizes, and initialize automatically.
- Passing strings to functions can be done by passing the character array. The array contains the characters of the string which gets passed to the function through this mechanism.
Similar Posts
Checkout more C Tutorials here.
I hope You liked the post 👍. For more such posts, 📫 subscribe to our newsletter. Try out our free resume checker service where our Industry Experts will help you by providing resume score based on the key criteria that recruiters and hiring managers are looking for.
FAQ
What are strings in C programming?
Strings in C programming are arrays of characters terminated by a null character (‘\0’). They are used to represent and manipulate text data efficiently.
Why use C strings?
C strings are used because they provide a convenient way to work with text data, allowing for operations like concatenation, comparison, and manipulation.
How to take string in C?
To take a string input in C, you can use functions like scanf()
with %s
format specifier or fgets()
.
How to print string in C?
To print a string in C, you can use printf()
with %s
format specifier. For example: printf("String: %s\n", str);
where str
is the string variable to be printed.