Table of Contents
ToggleThe goto statement allows unconditional jumping to any point in a C function. While powerful, goto can create confusing code if overused.
In this article, we will see the syntax and working of Goto Statement In C Programming and how to apply Goto judiciously in C, to simplify logic when needed while favouring structured programming practices. So, let’s get started.
What are Goto Statements in C Programming?
The goto statement is a control flow instruction that can be found in several programming languages, including C. It allows a program to jump to a specified label within a function unconditionally. This label marks a place in the code where execution is to continue.
Imagine you’re reading a choose-your-own-adventure book. A goto statement is like an instruction to skip to a certain page number, where the story continues from a new point.
In programming terms, the goto statement can be both powerful and dangerous. It’s powerful because it gives you direct control over the flow of execution. It’s dangerous because if used without care, it can make the program flow hard to follow and maintain, leading to what is known as “spaghetti code.“
Goto Syntax in C
The syntax of the goto statement in C is straightforward. You have two main components:
- goto statement
- target label.
Here’s how it looks:
goto label;
...
label:
statement;
The goto keyword is followed by a label name, which is then followed by a semicolon. Elsewhere in your code, you define the label followed by a colon and then the statement where the execution should jump to.
Flow Diagram of goto Statement in C
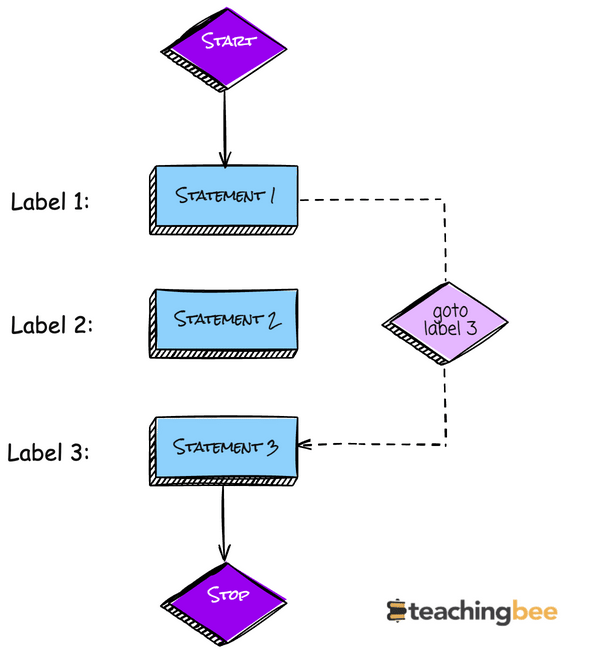
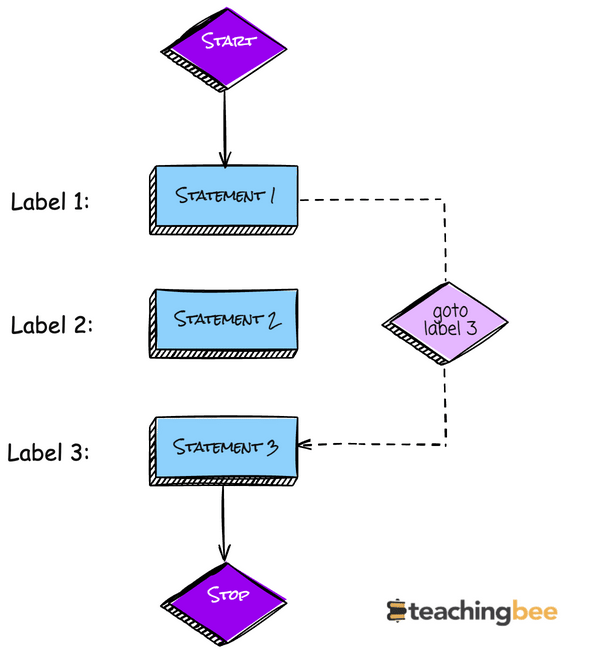
In the flowchart above, there are 3 labels defined: Label 1, Label 2, and Label 3. When Statement 1 is reached by the compiler, the program flow will be redirected to Label 3 due to the use of the goto Statement in C. Label 2 will not be executed.
How to use Goto in C?
To understand the basic operation of the goto statement, let’s look at a simple code snippet:
// Basic Operation and Flow Control with Goto
#include <stdio.h>
int main() {
int age = 20;
// Check if the person is an adult
if (age >= 18) {
goto adult;
}
// If the person is not an adult, print a message
printf("You are not an adult.\n");
goto end;
adult:
// If the person is an adult, print a message
printf("You are an adult.\n");
end:
// End of the program
return 0;
}
Output
You are an adult.
In this example, if the age variable is 18 or over, the program jumps to the adult label, skipping the “You are not an adult” message.
After printing “You are an adult” the program jumps to the end label, which leads to the end of the program.
The goto statement can be useful in handling errors and breaking out deeply nested loops. However, it should be used sparingly. Overusing goto can lead to code that’s hard to read and maintain. It’s often better to use structured control flow constructs like loops and functions, which we’ll discuss in other sections.
Remember, with great power comes great responsibility. The goto statement, while a tool in your programming toolkit, should be used judiciously and with clear intent.
Goto Statement In C with Examples
Let’s see different examples and patterns where using Goto statement can be very beneficial.
Common Patterns For Using Goto In C
Pattern 1: Error Handling
One of the most justified uses of goto is to handle errors in a series of operations that require cleanup before exiting a function. Here’s how you can do it:
// Error Handling with Goto Statements In C
#include <stdio.h>
#include <stdlib.h>
void *allocate_memory(size_t size) {
void *p = malloc(size);
if (p == NULL) {
goto error;
}
// Initialization or further processing goes here
return p;
error:
// Handle error, log it, and clean up if necessary
fprintf(stderr, "Failed to allocate %zu bytes of memory.\n", size);
// Return NULL to indicate failure
return NULL;
}
int main() {
void *memory_block = allocate_memory(1024);
if (memory_block == NULL) {
// Error handling if memory allocation failed
exit(EXIT_FAILURE);
}
// Use the allocated memory
// ...
// Clean up
free(memory_block);
return 0;
}
In this example, if memory allocation fails, the program jumps to the error label, where an error message is printed, and NULL is returned.
Pattern 2: Breaking from Nested Loops
Another common use case for goto is to break out from nested loops when a certain condition is met, which would be cumbersome with the usual loop control statements.
// Breaking from Nested Loops With Goto Statement In C
#include <stdio.h>
int main() {
int i, j;
int found = 0; // Flag to indicate if the value is found
for (i = 0; i < 5; i++) {
for (j = 0; j < 5; j++) {
printf("Checking: i=%d, j=%d\n", i, j);
if (i == 2 && j == 3) { // Suppose we need to stop here
found = 1; // Set the flag
goto end_search; // Break out of both loops
}
}
}
end_search:
if (found) {
printf("Found the value at i=%d, j=%d\n", i, j);
} else {
printf("Value not found.\n");
}
return 0;
}
Output
Checking: i=0, j=0
Checking: i=0, j=1
Checking: i=0, j=2
Checking: i=0, j=3
Checking: i=0, j=4
Checking: i=1, j=0
Checking: i=1, j=1
Checking: i=1, j=2
Checking: i=1, j=3
Checking: i=1, j=4
Checking: i=2, j=0
Checking: i=2, j=1
Checking: i=2, j=2
Checking: i=2, j=3
Found the value at i=2, j=3
In this code, when the condition i == 2 && j == 3 is true, the goto statement is executed, causing the program to jump to the end_search label, effectively breaking out of both loops and allowing us to handle the post-search logic in one place.
Pros of Using Goto Statements
Some of the advantages of using Goto statements In C are:
1. Code Simplification in Complex Control Flows
In complex control flows, especially those with many conditions, goto can simplify the code by avoiding deeply nested if statements.
2. Performance Optimization
In performance-critical sections of code, goto can sometimes provide a minor performance benefit by reducing the overhead of function calls or loop checks.
3. Historical Code Maintenance
In legacy systems, goto may be present and necessary to maintain. Understanding and using goto can be essential when dealing with such systems.
4. Mimicking Finally Blocks
C doesn’t have finally blocks like Java or C#. goto can be used to mimic this behavior for resource cleanup.
// Mimicking Finally Blocks With Goto
void perform_critical_task() {
// Resource acquisition
// ...
if (/* error condition */) {
goto cleanup;
}
// More operations
cleanup:
// Resource cleanup similar to a finally block
}
5. State Machine Implementation
goto can be useful in implementing state machines, where each state is labelled, and the program jumps between states based on events or conditions.
// State Machine using Goto statements
void state_machine() {
goto start_state; // Initialize state machine
start_state:
// Handle start state
if (/* condition to move to next state */) {
goto next_state;
}
// ...
next_state:
// Handle next state
// ...
}
6. Interrupt Handling Routines
In low-level programming, such as writing interrupt service routines, goto can be used for flow control to quickly jump to error handling or cleanup sections.
Each of these advantages comes with the caveat that goto should be used judiciously. The goal is always to write code that is efficient, clear, and maintainable.
Cons of Goto Statement In C
Let’s see some of the disadvantages of using goto statement in C.
1. Can Lead to Spaghetti Code
Overuse of goto can lead to code that is difficult to read and maintain, often referred to as “spaghetti code.”
// Hypothetical example of bad goto usage
goto part3;
part1:
// ...
goto part2;
part3:
// ...
goto part1;
part2:
// ...
This snippet is an exaggerated example of how goto can create a confusing flow that’s hard to follow.
2. Makes Code Less Modular
Using goto can make functions less modular and reusable, as the flow of the program becomes tightly coupled with the goto logic.
When to Use Goto Statement
The key to using goto effectively is balance. It should be used when it clearly simplifies the code without sacrificing structure and readability.
Use goto for:
- Centralized error handling and resource cleanup.
- Exiting from multiple nested loops when necessary.
Avoid goto when:
- The same outcome can be achieved with structured programming constructs like loops and functions.
- It leads to jumps that make the code hard to follow.
Alternatives Of Goto Statement
Let’s explore how we can replace goto with more structured constructs.
Structured Programming Constructs
Structured programming is a paradigm aimed at improving the clarity, quality, and development time of a computer program by making extensive use of subroutines, block structures, for loops, and while loops.
For instance, a goto used for looping can be replaced with a while loop:
// Using goto for looping
int counter = 0;
start_loop:
if (counter < 10) {
printf("%d ", counter);
counter++;
goto start_loop;
}
// Equivalent while loop
int counter = 0;
while (counter < 10) {
printf("%d ", counter);
counter++;
}
Loop and Control Statements as Substitutes
Loop and control statements are the backbone of structured programming. They offer clear ways to handle repetitive tasks and decision-making processes without resorting to goto.
For example, break and continue statements can manage flow control inside loops effectively:
// Flow Control using Goto
#include <stdio.h>
int main() {
for (int i = 0; i < 10; i++) {
if (i == 5) {
goto end_loop;
}
printf("%d ", i);
}
end_loop:
return 0;
}
// Using break to achieve the same result
#include <stdio.h>
int main() {
for (int i = 0; i < 10; i++) {
if (i == 5) {
break;
}
printf("%d ", i);
}
return 0;
}
Refactoring Code to Avoid Goto
Refactoring is the process of restructuring existing computer code without changing its external behaviour. It’s often used to remove goto statements by introducing functions or changing the logic flow.
Consider a goto used for error handling:
// Using goto for error handling
if (error_condition) {
goto handle_error;
}
// Normal code execution
// ...
handle_error:
// Error handling code
This can be refactored into a function for better structure:
// Refactored into a function
void handleError() {
// Error handling code
}
int main() {
if (error_condition) {
handleError();
return -1;
}
// Normal code execution
// ...
return 0;
}
By refactoring, we encapsulate the error handling in its own function, which can be reused and easily tested.
Key Takeaways
- Goto provides an unconditional jump to a labelled point in code.
- It can simplify logic in complex control flows and optimizations.
- Overuse leads to an unmaintainable spaghetti code.
- Apply goto judiciously for focused use cases.
- Favour structured programming constructs when possible.
Checkout more C Tutorials here.
I hope You liked the post ?. For more such posts, ? subscribe to our newsletter. Try out our free resume checker service where our Industry Experts will help you by providing resume score based on the key criteria that recruiters and hiring managers are looking for.
FAQs
Is goto bad practice in C?
Overusing goto is considered bad practice as it can create convoluted spaghetti code. However, limited usage in focused areas can be justified.
When should I use goto in C?
Goto can be used for centralized error handling, breaking from nested loops, and other cases where it clearly simplifies logic without sacrificing structure.
How can I avoid using goto in C?
Using structured constructs like loops, if/else blocks, switch statements, functions and proper modularization can remove the need for most usages of goto.
What are the alternatives to goto in C?
Alternatives include while/for loops, if/else conditionals, break/continue, switch/case, functions, and refactoring code. With proper design, goto is rarely necessary