Table of Contents
ToggleWhat is a Union in C?
A union in C is a user defined data type that allows you to store different data types in the same memory location. Unlike structures, where each member has its own memory space, all members of a union share the same memory space. This makes unions efficient for situations where you need to represent different types using the same memory block. A union can include multiple members but only one member will be there that can store a value at any given time.
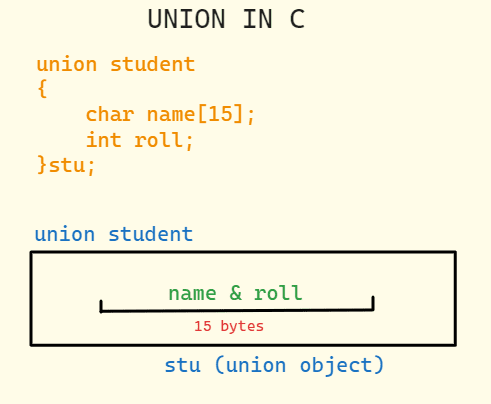
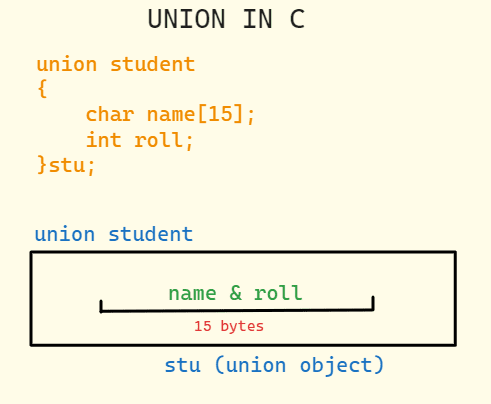
Memory Allocation of Union in C
One of the key aspects of unions is their memory allocation. The size of a union is determined by the size of its largest member. This is because all members share the same memory space, and the union needs to be large enough to accommodate the largest data type.
Here’s an example to illustrate how the size of a union is calculated:
#include <stdio.h>
union ExUnion {
int intnum;
float floatnum;
char stringVal[20];
};
int main() {
union ExUnion ex;
printf("Size of ExampleUnion: %lu bytes\n", sizeof(ex));
return 0;
}
Output
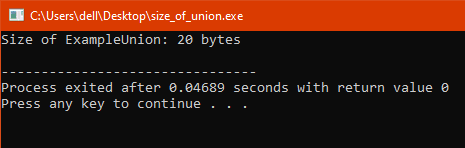
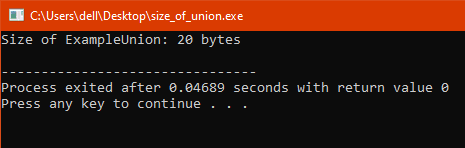
Syntax of Union in C
The syntax of a Union can be divided into three parts:
- Union declaration
- Union variable
- Accessing / Initializing union members
1. Union Declaration
union union_name {
// Member 1
data_type1 member1;
// Member 2
data_type2 member2;
// ...
// Member N
data_typeN memberN;
};
2. Union Variable
With Union declaration
union union_name {
// Member 1
data_type1 member1;
// Member 2
data_type2 member2;
// ...
// Member N
data_typeN memberN;
} variable_name;
After Union declaration
union union_name variable_name;
3. Accessing / Initializing Union Members
variable_name.member1 = value1;
variable_name.member2 = value2;
// ...
variable_name.memberN = valueN;
Union Program in C
Write a program in C to store and manipulate information about different shapes. The information to be stored includes either the radius of a circle, the side length of a square, or the base and height of a triangle. Use a union to efficiently represent these different types of shapes in a single data structure.
Algorithm
- Define a union named “Shape” with three members: “radius” for a circle, “sideLength” for a square, and a nested struct containing “base” and “height” for a triangle.
- Declare a variable of type “Shape” named “myShape”.
- Then initialize the “radius” member to represent a circle.
- Print information about the circle.
- Change the shape type to a square by updating the “sidelength”.
- Print information about the square.
- Change the shape type to a triangle by updating the “base” and “height”.
- Print information about the triangle.
Source Code
#include <stdio.h>
union Shape {
float radius;
float sideLength;
struct {
float base;
float height;
} triangle;
};
int main() {
union Shape myShape;
myShape.radius = 5.0;
printf("Shape Type: Circle\n");
printf("Radius: %.2f\n", myShape.radius);
myShape.sideLength = 4.0;
printf("\nShape Type: Square\n");
printf("Side Length: %.2f\n", myShape.sideLength);
myShape.triangle.base = 3.0;
myShape.triangle.height = 6.0;
printf("\nShape Type: Triangle\n");
printf("Base: %.2f\n", myShape.triangle.base);
printf("Height: %.2f\n", myShape.triangle.height);
printf("\nSize of Shape: %lu bytes\n", sizeof(myShape));
return 0;
}
Output
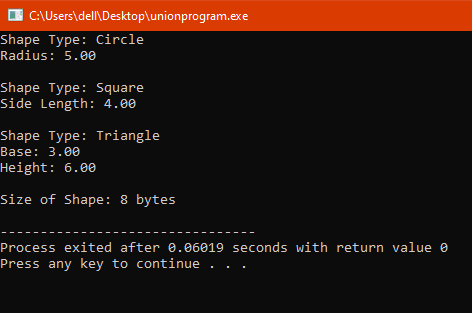
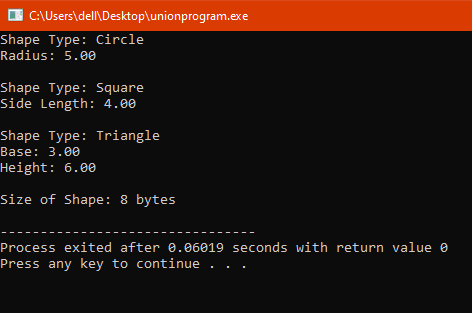
Advantages of Union in C
- Unions allow multiple members to share the same memory space. This enables efficient memory usage.
- Unions offer versatility by enabling a single variable to represent different data types.
- Accessing a member of a union involves direct access to shared memory, which can be more memory-efficient compared to maintaining separate variables.
Disadvantages of Union in C
- Unions can only hold one member’s value at a time. If there is a need to store and manipulate multiple types of data simultaneously, unions may not be the most suitable choice.
- Since all members share the same memory space, accessing a member with an incorrect type can result in undefined behavior.
Conclusion
In the C programming language, a union is a user-defined data type that allows different data types to be stored in the same memory location. It offers a powerful and efficient way to work with different data types in a single memory location. The size of a union is determined by the size of its largest member. By understanding their syntax and applications, you can enhance your ability to write flexible and memory-efficient code. Happy Learning!
I hope You liked the post 👍. For more such posts, 📫 subscribe to our newsletter. Try out our free resume checker service where our Industry Experts will help you by providing resume score based on the key criteria that recruiters and hiring managers are looking for.
FAQ
Can a union have a member that is a structure?
Yes, a union can have a member that is a structure. This is known as a nested union or a union within a structure.
union NestedUnion {
int intValue;
struct {
float floatValue;
char stringValue[20];
} nestedStruct;
};
Can unions be used for type casting in C?
Yes, unions can be used for type casting in C, allowing you to reinterpret the bits of one type as another. However, caution is required to ensure that the conversion is valid and does not violate type safety.
When should I use a union in my C program?
Unions are useful when you need to represent different types of data in a memory-efficient manner, especially when only one type is active at a given time. They are commonly used in scenarios involving variant types, binary data, and efficient memory usage.
How do unions differ from structures in C?
While structures allow multiple members to have their own memory space, unions share the same memory space for all members. Additionally, unions can only store the value of one member at a time, making them suitable for scenarios where different types of data need to be represented interchangeably.