Table of Contents
ToggleIntroduction
An API is an application programming interface. It’s a set of rules that controls how apps or devices interact with one another. APIs allow developers to communicate with the data. This makes them more user-friendly and less complicated. REST APIs have to be well-designed. If they aren’t, developers may find it more difficult than helpful. To provide the best service possible to clients, you must follow the REST API with java best-practices.
What is Rest API ?
RESTful also known as Representational State Transfer is designed for existing protocols. Although REST can use almost any protocol it is compatible with, it typically uses HTTP for Web APIs. This means that developers don’t need additional software or libraries in order to use a REST-based API design. Roy Fielding’s 2000 doctorate dissertation defined REST API Design. It is noted for its amazing flexibility. REST is able to handle multiple types, return different data formats, and even change structure with the right implementation of hypermedia. Data is not tied to resources or methods.
Best Practices for REST API With JAVA
To design high quality rest api with java it is imporatnt to follow some of the conventions and rest api best practices. Let’s look at some of the rest api best practices.
Making a Contract
The contract first approach is a method to develop and design API’s using a contract. In the case of REST APIs for java a contract-first approach refers to the process of creating a specification and then implementing your service. In your first attempt, this means that you create an OpenAPI document (or Swagger) and give it to your consumers/clients. Then, you implement your service against this specification. This approach has the following advantages.
- Easy integration: You and your customers will have to use the same contract when building their software. The integration will be seamless.
- Easier alignment and discussions: Making contract first will help to discuss with clients properly and help developers to align themselves with the requirements.
- Versioning: Each contract will have a version which will allow you to easily modify your API.
Say Hello To Json
REST APIs with java should be able to accept JSON requests payloads, and can also send JSON replies. JSON is a standard for data transfer. Nearly all networked technologies can use it. JavaScript has built in methods to encode or decode JSON through the Fetch HTTP API or another HTTP client. JSON can be decoded by server-side technology using libraries that don’t require much work.
There are other methods of data transfer. Frameworks do not support XML. However, they can transform the data to be usable. The client-side can’t handle this data as easily, especially in web browsers. It is a lot more work than just normal data transfers.
When we want to send files, form data can be useful for sending data. We don’t need any form data to send text and numbers. However, most frameworks support JSON transfer. The client can get the JSON directly from the framework. It’s the easiest and most convenient way.
Using HTTP Methods Effectively
When you think REST API with java, think HTTP. The HTTP request methods you need to use are important.The most frequently-used HTTP verbs or methods are DELETE, GET, GET, PUT and PATCH. These can be used to create (read, update, delete, or CRUD) operations. There are many other verbs that are used less frequently, like OPTIONS, and HEAD which are the most used of those less frequent methods.
Below is a table listing the recommended return value of the primary HTTP method in conjunction with the resource URLs.
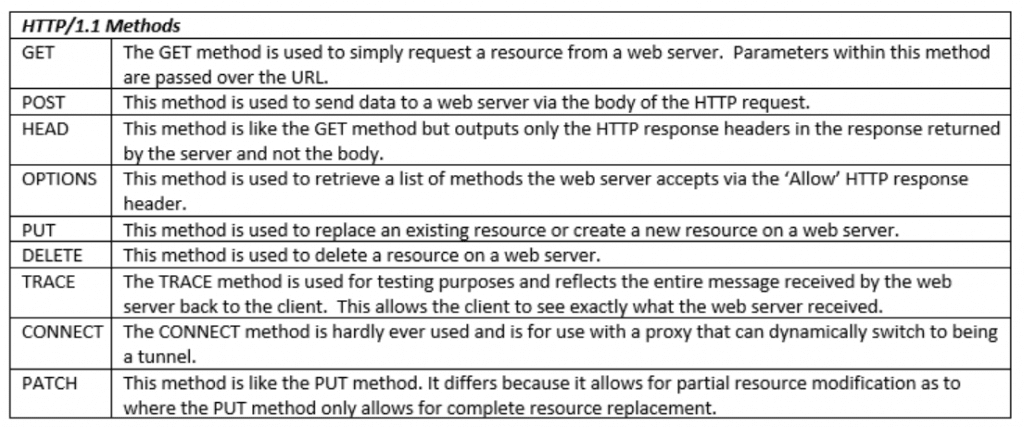
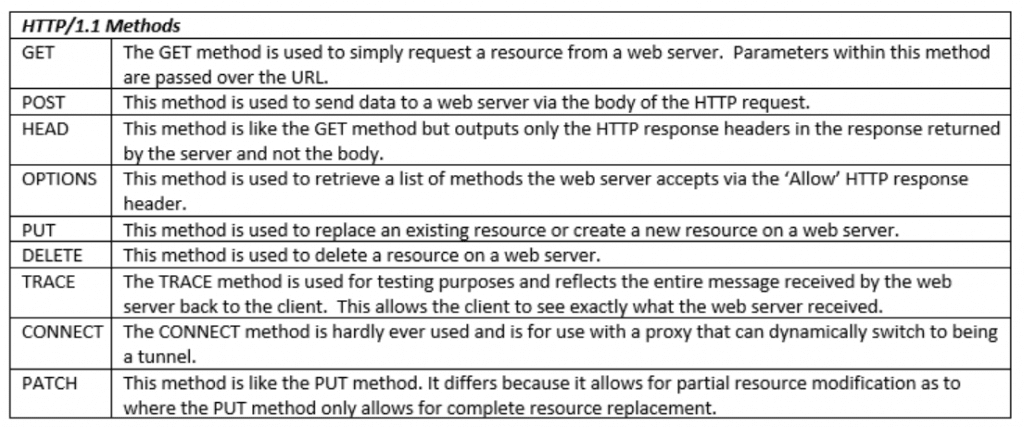
Getting Most Out Of HTTP Response Status
When you execute an operation, it is very important that you return the correct http response status.
If you are unable to find a resource, do not throw an exception. Instead, send the appropriate response codes in your response message like 404 or send back a 500 code if there is a server error.
These are the standard status codes defined by HTTP that can be used for a client to relay the result of their request. There are five main categories of status codes.
Response Code Category | Desciption |
1xx | Informational — Communicates transfer protocol level information. |
2xx | Success — Indicates that the client received their request successfully. |
3xx | Redirection — Indicates that the client needs to take further action to fulfill their request. |
4xx | Client Error — This category of error codes points fingers at clients. |
5xx | Server Error — The server assumes responsibility for these error codes. |
Documenting
Clear and detailed documentation is necessary. The Rest API with java definition often determines how documentation is created. You must ensure that people with less experience or little knowledge can understand the documentation.
Complete documentation is required to help users learn security, authentication, as well as error management. Additionally, it includes engaging tutorials, guides, as well easy-to follow resources. Users will find it much easier to use your API when there is extensive documentation.
Versioning
This practice allows developers to make changes to data structures and actions. If your project grows in time and size, you can work with multiple API versions. The benefit of this is that developers can make more enhancements to their service and hold on to those API users who refuse to change or are slow to accept new changes.
We have mixed views on whether it is better to include an API version in URLs or a header. Academically, it should go in the header. The REST API for java URL must contain the version. This allows the search to be performed across all versions of the browser, ensuring a seamless development experience.
APIs are usually unstable and can change frequently. Although it is impossible to avoid the possibility of change, you should consider the best ways to manage the change. Many APIs recommend that you plan and declare a depreciation for each month.
Types of versioning methods:
- URI versioning
- Request Param versioning
- Header versioning
- Media Type versioning
Take a look at Error Handling
Every API user should be able to recognize and avoid errors. This returns the HTTP response codes, which describe the nature and extent of the error. API maintainers have ample data to analyze the issue and determine its source.It is best to leave the errors unmanaged in order to maintain a system that is free from errors. API customers are responsible for handling errors. These basic error HTTP status codes are:
- 404 not found – This means that no resources are available.
- 403 forbidden – This means that an unauthorized user is not allowed to use a resource, even after user has been verified.
- 401 Unauthorized – This means that the user cannot use a resource. It will usually return if a user doesn’t get verified.
- 400 Bad Requests – This is a sign that client-side input failed to be validated or documented.
- 503 Service Not Available – This is a sign that an unexpected and unplanned action occurred on the server’s side.
- 502 Bad Gateway – This is an indication that a crucial server has not responded to your request.
- 500 Internal Server Problem – This is a common server error.
Getting Friendly With Open API Documentation
OpenAPI Specification like swagger defines a standard language-agnostic interface with REST API for java. This allows both humans (and computers) to discover and comprehend the service’s capabilities without needing to have access to source code or documentation. The service can be understood and accessed by the consumer with little to no implementation logic when it is well defined.
OpenAPI can then used by documentation generation software to display the API, code generator tools to generate clients and servers using various programming languages, testing instruments, and many more.
Security First
It is one of rest api best practices to use current security frameworks such TLS or SSL when creating rest API with java . SSL certificates are able to establish a secure connection by providing a private as well as public key. Without an SSL certificate, you can’t be certain that you are properly protecting sensitive information such as medical or financial data.
TLS (Transport Layer Security) is SSL’s latest version, which provides better security and protection. Regular testing is a key component of API security. These two tests can be used:
- Penetrating Test – This test determines the vulnerability of APIs to a cyber attack. The tester searches for vulnerabilities that might be used by hackers.
- Fuzz Testing – It is a test that checks how APIs respond when there is an invalid or unnecessary input. This test helps to find flaws and errors in the code.
Handling Large Data
The databases behind a REST API with java can get very large .Sometimes, the amount of data in a REST API can be so large that it is not possible to return all of it at once. This is why we need to filter items.
We need ways to paginate the data so we can only return a limited number of results. We don’t want too many requests at once, which can lead to over-complication.
Filtering, pagination and other features can increase performance by reducing server resources. These features are increasingly important and is one of rest api best practices to include them when designing rest api with java.
Caching
Caching is better than asking for data multiple times. Caching offers the user faster access to data. However, users could also be able to access outdated data. Also, outdated data can lead to problems while fixing in production environments.So proper cache techniques need to be incorporated in the design of rest api for java.
Final Words
To design high-quality RESTAPIs, it is important to follow web conventions and standards. The rest api best practices outlined above can help you meet your development targets using REST API with java . These best practices can be hard to follow. A platform that manages APIs can make it easy to create successful APIs even if you don’t know much about coding.
If you have any questions about Rest Api for java, please join our newsletter and ask a question! We’re happy to help you learn more.