Table of Contents
ToggleAccess modifiers or access specifiers in Java control access to classes, methods, and member variables. They determine the visibility and accessibility of class members. Choosing the right access level is vital for encapsulation and API design.
In this article we will understand the four access specifiers along with their visibility, usage and best practices for access control.
What Is Access Specifier In Java?
Access Modifiers Or Access specifiers in Java are keywords that define the visibility or accessibility of classes, methods, and fields within a program.They determine whether these members can be accessed from outside the class or only within the class itself. They control how different parts of a Java program can interact with and access one another.
Different Types Of Access Specifiers In Java
Java provides four Access Specifiers/Access Modifiers, each with its own level of visibility. The four access specifiers in Java are:
- public
- private
- protected
- Default (Package-Private)
Let’s understand each of these access specifiers/modifiers in detail.
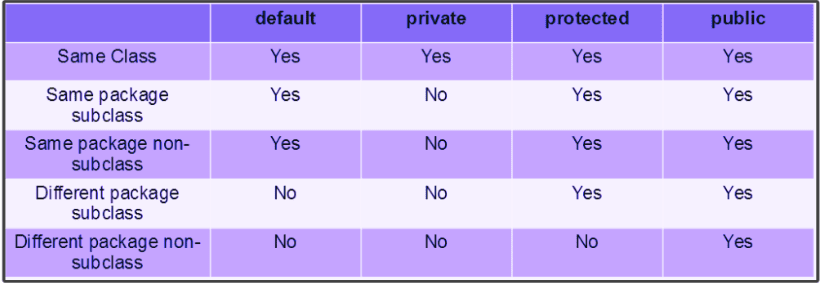
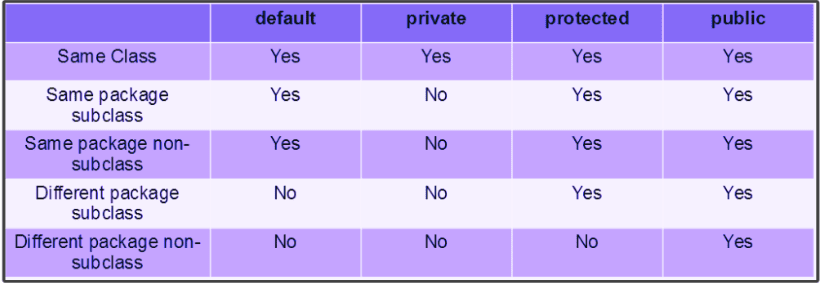
public Access Specifier In Java
The public
access specifier in Java is the most permissive. It allows a class, field, or method to be accessed from any other class, regardless of the package. Members declared as public
become part of the class’s public interface.
Characteristics
- Accessible Anywhere: Public members are accessible from any class, regardless of the package.
Use Cases and Considerations
- Use
public
when a member needs to be accessed from any part of the program. - Suitable for creating a well-defined and widely used API.
public class PublicExample {
// Public field accessible from any class
public int publicVar;
// Public method accessible from any class
public void publicMethod() {
System.out.println("This is a public method.");
}
}
public class AnotherClass {
public static void main(String[] args) {
// Creating an instance of PublicExample
PublicExample publicExampleObject = new PublicExample();
// Accessing the public field from AnotherClass
publicExampleObject.publicVar = 42;
// Calling the public method from AnotherClass
publicExampleObject.publicMethod();
// Printing the value of the public field
System.out.println("Value of publicVar: " + publicExampleObject.publicVar);
}
}
In this example, PublicExample
defines a public class with a public field and method, and AnotherClass
demonstrates the accessibility of these public members from another class. This illustrates the use of the public
access specifier in Java.
private Access Specifier In Java
The private
access specifier in Java is the most restrictive. Members declared as private
are accessible only within the same class.
Characteristics
- Accessible Within the Class Only:
private
members are accessible only within the class in which they are defined.
Use Cases and Considerations
- Hiding Implementation Details: Use
private
when you want to hide implementation details and ensure a high level of encapsulation.
public class PrivateAccessExample {
private int privateVar;
public PrivateAccessExample(int initialValue) {
this.privateVar = initialValue;
}
public int getPrivateVar() {
return privateVar;
}
}
public class AnotherClass {
public static void main(String[] args) {
// Creating an instance of PrivateAccessExample
PrivateAccessExample example = new PrivateAccessExample(42);
// Attempting to access privateVar directly (will result in a compilation error)
// Uncommenting the line below will result in a compilation error
// System.out.println("Value of privateVar: " + example.privateVar);
// Accessing privateVar using a public method
System.out.println("Value of privateVar using getPrivateVar: " + example.getPrivateVar());
}
}
In this example:
- The
AnotherClass
attempts to access the private variableprivateVar
directly, which will result in a compilation error. - Access to
privateVar
is then demonstrated using a public method (getPrivateVar
) as the proper way to access private members from outside the class.
protected Access Specifier In Java
The protected
access specifier in Java allows a member (field or method) to be accessed within its own package and by subclasses, whether they are in the same or different packages. It provides a middle ground between the unrestricted public
access and the more restricted package-private (default) and private
access.
This level of access is useful when you want to share certain functionalities with subclasses while still maintaining some level of encapsulation.
Characteristics
- Accessible Within Package: Members declared as
protected
are accessible within the same package. - Accessible by Subclasses:
protected
members can be accessed by subclasses, whether in the same or different packages.
Use Cases and Considerations
- Sharing Functionality Among Subclasses: Use
protected
when sharing certain functionalities with subclasses.
// File: MyBaseClass.java
public class MyBaseClass {
// Protected field accessible within its own package and by subclasses
protected int protectedVar;
// Protected method accessible within its own package and by subclasses
protected void protectedMethod() {
System.out.println("This is a protected method.");
}
}
// File: MyDerivedClass.java
public class MyDerivedClass extends MyBaseClass {
public static void main(String[] args) {
// Creating an instance of MyDerivedClass
MyDerivedClass derivedClassObject = new MyDerivedClass();
// Accessing the protected field from MyDerivedClass
derivedClassObject.protectedVar = 42;
// Calling the protected method from MyDerivedClass
derivedClassObject.protectedMethod();
// Printing the value of the protected field
System.out.println("Value of protectedVar: " + derivedClassObject.protectedVar);
}
}
In this example, MyBaseClass
defines a class with a protected field and method. MyDerivedClass
extends MyBaseClass
and demonstrates the accessibility of these protected members.
The protected field and method are accessible within the same package and by subclasses, showcasing the behaviour of the protected
access specifier in Java.
Default Access Specifier In Java
When no access specifier is specified (default), the access level is package-private. This means that members are accessible only within their own package.
Characteristics
- Accessible Within Package Only: Members with default access are accessible only within the same package.
Use Cases and Considerations
- Encapsulation Within a Package: Use default access specifier when restricting access to members within the same package.
// File: DefaultAccessExample.java
class DefaultAccessExample {
// Default (package-private) field
int defaultVar;
// Default (package-private) method
void defaultMethod() {
System.out.println("This is a default method.");
}
}
// File: AnotherClass.java
public class AnotherClass {
public static void main(String[] args) {
// Creating an instance of DefaultAccessExample
DefaultAccessExample defaultExampleObject = new DefaultAccessExample();
// Accessing the defaultVar field directly (since it is package-private)
defaultExampleObject.defaultVar = 20;
// Accessing the defaultMethod directly (since it is package-private)
defaultExampleObject.defaultMethod();
// Printing the value of the defaultVar field
System.out.println("Value of defaultVar: " + defaultExampleObject.defaultVar);
}
}
In this above example, Both defaultVar
and defaultMethod
are used in AnotherClass without any compilation errors as both are placed in same package, showcasing the default access within the same package.
Key Takeaways
- There are four access specifiers in Java – public, private, protected and default (package-private)
- public provides visibility everywhere, private only within the class
- protected allows access in the same package and subclasses
- default enables access only within the same package
I hope You liked the post 👍. For more such posts, 📫 subscribe to our newsletter. Try out our free resume checker service where our Industry Experts will help you by providing resume score based on the key criteria that recruiters and hiring managers are looking for.
FAQ
What are the 12 modifiers in Java?
In Java, the commonly used modifiers include public
, abstract
, final
for classes; public
, private
, protected
, default
, static
, and final
for fields; and public
, private
, protected
, default
, static
, abstract
, final
, synchronized
, and native
for methods.
What is the significance of access specifiers in Java?
Access specifiers in Java control the visibility of classes, fields, and methods. They define the scope of access for members, determining which parts of the program can interact with them. Proper use of access specifiers enhances encapsulation, security, and modularity in Java programs.
When should I use the public
access specifier?
Use the public
access specifier when you want a class, field, or method to be accessible from any part of the program. This is useful for creating a well-defined and widely used API. Public members become part of the class’s public interface.
What is the difference between protected
and default
(package-private) access specifiers?
Both protected
and default access specifiers allow access within the same package. However, protected
additionally allows access by subclasses, even if they are in different packages. protected
is often used when sharing functionality among subclasses, while default access is limited to the same package.