Table of Contents
ToggleInheritance is a powerful feature in object-oriented programming languages like Java that allows classes to inherit attributes and behaviours from parent classes. This enables code reuse and establishing class hierarchies.
By default, Java only allows a class to inherit from one direct parent class. However, some languages support multiple inheritance, where a class can inherit from multiple parent classes.
While multiple inheritance provides greater flexibility, it can also introduce a particular issue known as the “diamond problem” ambiguity.
In this article, we will cover:
- What is multiple inheritance?
- What is diamond problem in Java?
- Why Java doesn’t support multiple class inheritance?
- How to solve Diamond Problem in Java?
But discussing diamond problem in java.Let’s look into some basics first.
Inheritance in Object-Oriented Programming
Inheritance is one of the core principles of object-oriented programming (OOP). It allows a class to inherit properties (attributes) and methods (behaviours) from another class, referred to as its parent or superclass. This leads to a hierarchy of classes that share features and behaviours, promoting code reuse and making it easier to create and maintain complex systems.
Types Of Inheritance In OOPS
- Single Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
- Multiple Inheritance
- Hybrid Inheritance
Inheritance Example In Java
// Inheritance Example In Java
// Base class
class Animal {
public void eat() {
System.out.println("This animal eats food.");
}
}
// Derived class
class Dog extends Animal {
public void bark() {
System.out.println("The dog barks.");
}
}
// Usage
public class TestInheritance {
public static void main(String[] args) {
Dog myDog = new Dog();
myDog.eat(); // Inherited method
myDog.bark(); // Own method
}
}
In the above example, Dog
is a subclass of Animal
and inherits the eat
method. This demonstrates how a single class can be extended to reuse functionality.
Multiple Inheritance
Multiple inheritance allows a class to inherit features from more than one parent class. This can make the design of a program more flexible by combining different behaviours from multiple sources.
What Is Diamond Problem In Java?
The Diamond Problem In Java is an infamous complication in object-oriented programming that arises with multiple inheritance. This problem occurs when a class inherits from two classes that have a common base class.
This can lead to ambiguity about which base class methods and properties the derived class should inherit if both intermediate base classes have modified them.
Here’s a more detailed theoretical example to illustrate the Diamond Problem:
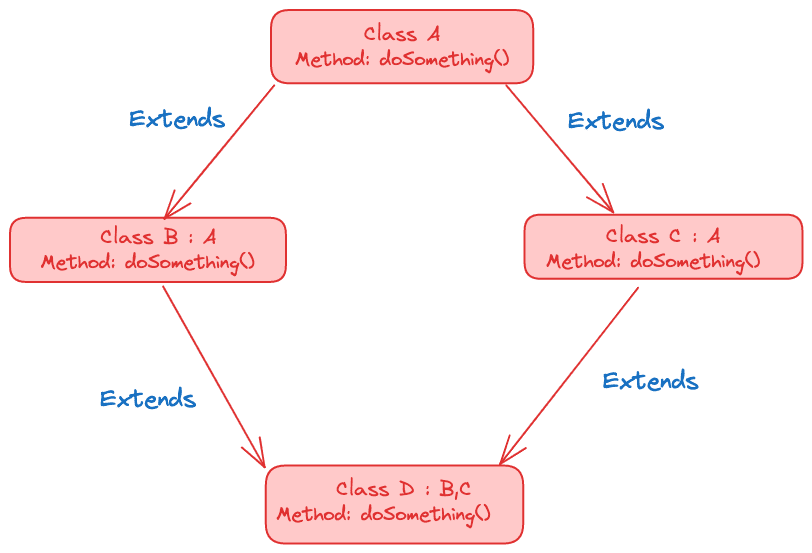
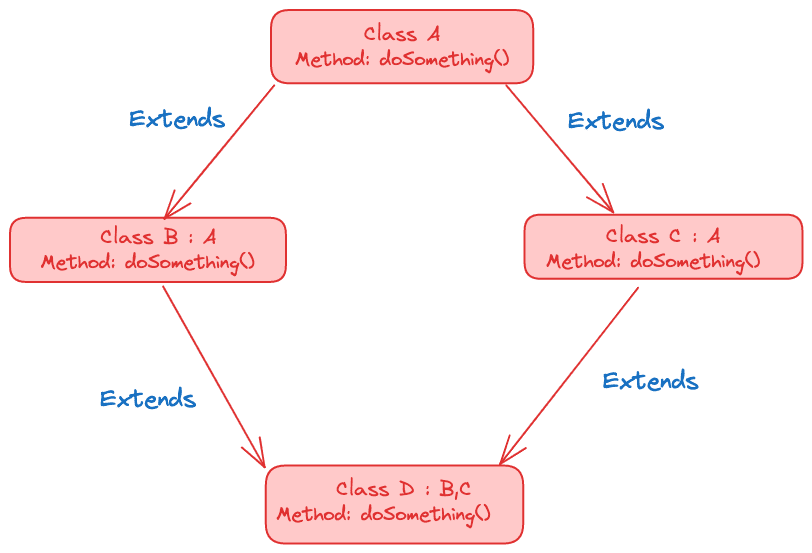
In this diamond-shaped class structure:
- Class A is the top-most base class with a method doSomething().
- Classes B and C are subclasses of A and may override the doSomething() method.
- Class D inherits from both B and C.
Why Doesn’t Java Support Multiple Inheritance?
Java was designed to avoid the complexity and ambiguity of multiple inheritance seen with the diamond problem. Here’s why:
Avoiding the Diamond Problem
Java’s single inheritance model prevents the ambiguity of multiple inheritance paths. Each class has one, and only one, superclass. There’s always a clear line from a child’s class up through its ancestry.
Simplicity and Maintainability
Single inheritance encourages a simpler and more maintainable class hierarchy. It enforces a straightforward relationship between classes, making it easier for developers to trace through the code and predict how objects will behave.
In Java, the Diamond Problem is averted by not allowing multiple-class inheritance. However, to still benefit from some aspects of multiple inheritance, Java provides several solutions and workarounds.
Solution Of Diamond Problem In Java
Using Interfaces
Java allows a class to implement multiple interfaces, which can define methods without their implementation (before Java 8) or with a default implementation (since Java 8). Classes can implement more than one interface, allowing them to inherit multiple sets of method signatures.
// Multiple Interface Inheritance
interface Flyable {
void fly();
}
interface Driveable {
void drive();
}
class FlyingCar implements Flyable, Driveable {
public void fly() {
System.out.println("Flying.");
}
public void drive() {
System.out.println("Driving.");
}
}
In this example FlyingCar implements multiple interfaces Flyable and Driveable and inherits there function signatures.
How To Solve Diamond Problem Using Default Methods in Java?
Java 8 introduced default methods in interfaces, enabling interfaces to provide an implementation that can be shared among multiple implementing classes.
When a class implements multiple interfaces that have conflicting default methods, Java forces the class to override the method and decide which interface’s default method to use or to provide a new one.
// Default Methods
interface FirstInterface {
default void perform() {
System.out.println("First");
}
}
interface SecondInterface {
default void perform() {
System.out.println("Second");
}
}
class MultipleInheritanceClass implements FirstInterface, SecondInterface {
// Overriding to resolve ambiguity
@Override
public void perform() {
FirstInterface.super.perform(); // Using FirstInterface's perform method
}
}
- In the abbove example, the class
MultipleInheritanceClass
implements bothFirstInterface
andSecondInterface
. Since both interfaces provide a default implementation of the same methodperform
, Java doesn’t know which version to use when the method is called on an instance ofMultipleInheritanceClass
. - To resolve this ambiguity,
MultipleInheritanceClass
explicitly overrides theperform
method. Within this overridden method, it specifies which version ofperform
it wants to use by callingFirstInterface.super.perform()
. This syntax explicitly tells Java to use theperform
method as defined inFirstInterface
. - If the class didn’t override the
perform
method, it would result in a compiler error because of the ambiguity regarding whichperform
method should be inherited.
Default methods can be overridden in implementing classes, allowing for customisation of behaviour.
// Overriding Default Methods
interface Vehicle {
default void start() {
System.out.println("Vehicle starting.");
}
}
class ElectricCar implements Vehicle {
// Overriding the default method for customization
@Override
public void start() {
System.out.println("ElectricCar starting silently.");
}
}
Key TakeAways
To Conclude:
- The diamond problem is ambiguity that arises when a class inherits from multiple parent classes that share a common base class.
- Java prohibits multiple class inheritance to avoid the diamond problem and keep the class hierarchy simple.
- Interfaces can be used for a limited form of multiple inheritance in Java. Classes can implement multiple interfaces.
- Default methods allow interfaces to provide implementations that can be reused or overridden by classes.
Checkout more Java Tutorials here.
I hope You liked the post ?. For more such posts, ? subscribe to our newsletter. Try out our free resume checker service where our Industry Experts will help you by providing resume score based on the key criteria that recruiters and hiring managers are looking for.
FAQ
Does Java support multiple inheritance?
No, Java does not support multiple inheritance between classes. Only single class inheritance is allowed.
Can an interface extend multiple interfaces in Java?
Yes, interfaces in Java can extend multiple parent interfaces using the extends keyword.
How are Java abstract classes different from interfaces?
Abstract classes can contain both abstract and concrete methods, while interfaces contain only abstract methods. Classes can extend only one abstract class but can implement multiple interfaces.
What is a mixin in Java?
A mixin is a class that provides certain functionality to be inherited by other classes without having to be the parent class. Mixins are used to emulate multiple inheritance in Java.