Table of Contents
ToggleIn this article, we will see how to calculate area of hexagon in Java. A hexagon is a six-sided polygon with equal sides, and calculating its area involves geometry and trigonometry. Let’s dive in.
Problem Statement
Given the side length of a regular hexagon, we need to calculate its area. The input will include the side length, and the output should be the area of the hexagon. For Example:
Input:
Side length of the hexagon: 5.0 units
Output:
Area of the hexagon: 64.95 square units (rounded to two decimal places)
Solution
What Is The Area Of A Hexagon?
Area of a Regular Hexagon Formula: The area (A) of a regular hexagon with side length “s” can be calculated using the formula:
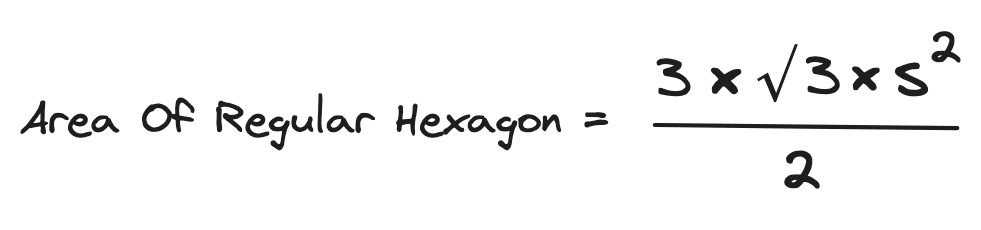
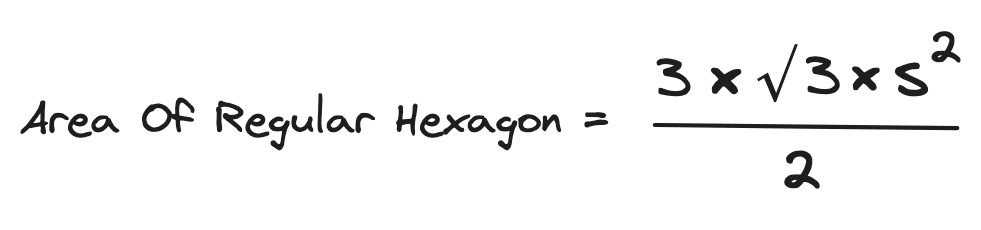
where “s” is the side length of the hexagon.
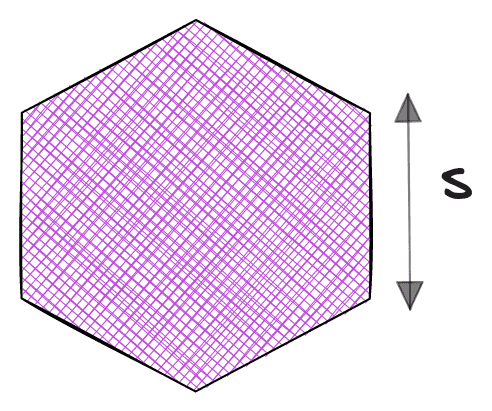
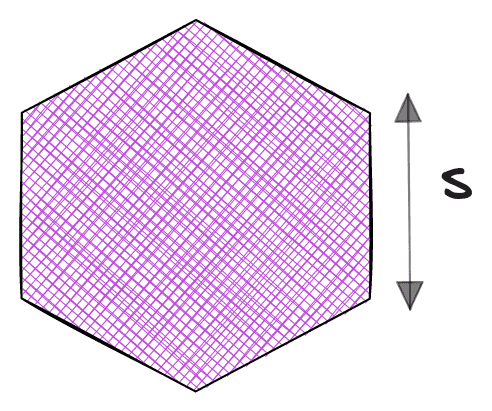
For example, let’s say we have a regular hexagon with a side length “s” of 6 units. We want to find its area.
- Putting the side length “s” into the formula: Area = (3√3/2) * (6²)
- Calculate 6², which is 36: Area = (3√3/2) * 36
- Calculate 3√3/2, which is approximately 2.598 (you can use a calculator for this): Area ≈ 2.598 * 36
- Multiply 2.598 by 36 to find area of hexagon : Area ≈ 93.528 square units
So, the area of a regular hexagon with a side length of 6 units is approximately 93.528 square units.
Algorithm
- Accept the side length of the hexagon as input.
- Calculate the area using the above area of a regular hexagon formula
- To calculate square root of three, use Math.sqrt() function provided in Math libraryin java
- Similarly, To get square of number use Math.pow()
- Display the calculated area.
Java Code Implementation
// Calculate Area of Hexagon in Java
import java.util.Scanner;
public class CalculateHexagonArea {
public static void main(String[] args) {
// Create a Scanner for user input
Scanner scanner = new Scanner(System.in);
// Prompt the user to enter the side length
System.out.print("Enter the side length of the hexagon: ");
double sideLength = scanner.nextDouble();
// Calculate the area of the hexagon
double area = (3 * Math.sqrt(3) * Math.pow(sideLength, 2)) / 2;
// Display the calculated area
System.out.printf("The area of the hexagon is: %.2f square units%n", area);
// Close the scanner
scanner.close();
}
}
Output
Enter the side length of the hexagon: 5.0
The area of the hexagon is: 64.95 square units
Key Takeaways
- The area of a regular hexagon can be calculated using the formula A = (3 * √3 * s^2) / 2, where “s” is the side length of the hexagon.
- Java provides the
Math.sqrt
function to calculate square roots and theMath.pow
function for exponentiation.
Similar Posts
Add Days To The Current Date in Java
Program to Calculate EMI in Java
Calculate Area of a Circle in Java
Checkout more Java Tutorials here.
I hope You liked the post ?. For more such posts, ? subscribe to our newsletter. Try out our free resume checker service where our Industry Experts will help you by providing resume score based on the key criteria that recruiters and hiring managers are looking for.
FAQ
Can I calculate the area of an irregular hexagon using the same formula?
No, the formula provided in this article is specific to regular hexagons, which have all sides of equal length and all angles of equal measure. For irregular hexagons, you would need to use a different approach based on the specific side lengths and angles.
Are there any specialized libraries or functions for geometric calculations in Java?
Java’s standard library (java.lang.Math
) provides most mathematical functions needed for geometric calculations. For more advanced geometry or trigonometry, you may consider using third-party libraries or frameworks designed for such purposes.