Table of Contents
ToggleThe Strategy Design Pattern is a behavioral pattern that enables selecting an algorithm’s runtime behaviour among a family of algorithms. It encapsulates each algorithm, allowing them to be interchangeable within that family.
The key objective is to separate the concerns of how a certain task is performed from the context in which it is used, promoting a more modular and flexible design.
What is Strategy Design Pattern?
The Strategy Design Pattern is a behavioral software design pattern that enables selecting an algorithm’s behavior at runtime. Instead of implementing a single algorithm directly, code receives run-time instructions as to which in a family of algorithms to use.
Consider an application that processes payments. Initially, you might support only one payment method, such as credit card payments. However, as the application grows, you need to support additional payment methods like PayPal, bank transfers, or cryptocurrencies.
Without the Strategy Pattern, you might find yourself adding multiple conditional statements throughout your code to handle each payment method differently, leading to a system that’s hard to maintain and extend.
class PaymentProcessor {
void processPayment(double amount, String method) {
if (method.equals("CreditCard")) {
// Process credit card payment
} else if (method.equals("PayPal")) {
// Process PayPal payment
}
// More conditions for other payment methods
}
}
This approach quickly becomes unwieldy and violates the Open/Closed Principle as new payment methods require modifications to the existing PaymentProcessor
class.
Solving the Issue with Strategy Design Pattern
The Strategy Pattern addresses this issue by defining a family of algorithms (in this case, payment methods), encapsulating each one, and making them interchangeable. The payment processing strategy can vary independently from clients that use it.
How to Implement Strategy Design Pattern?
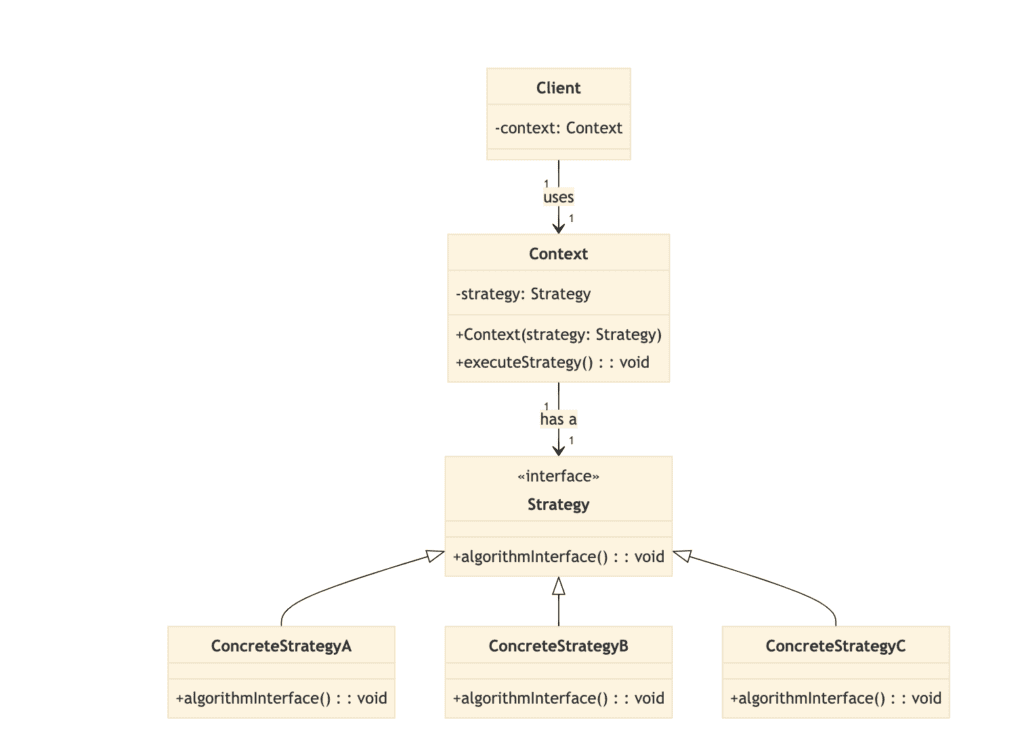
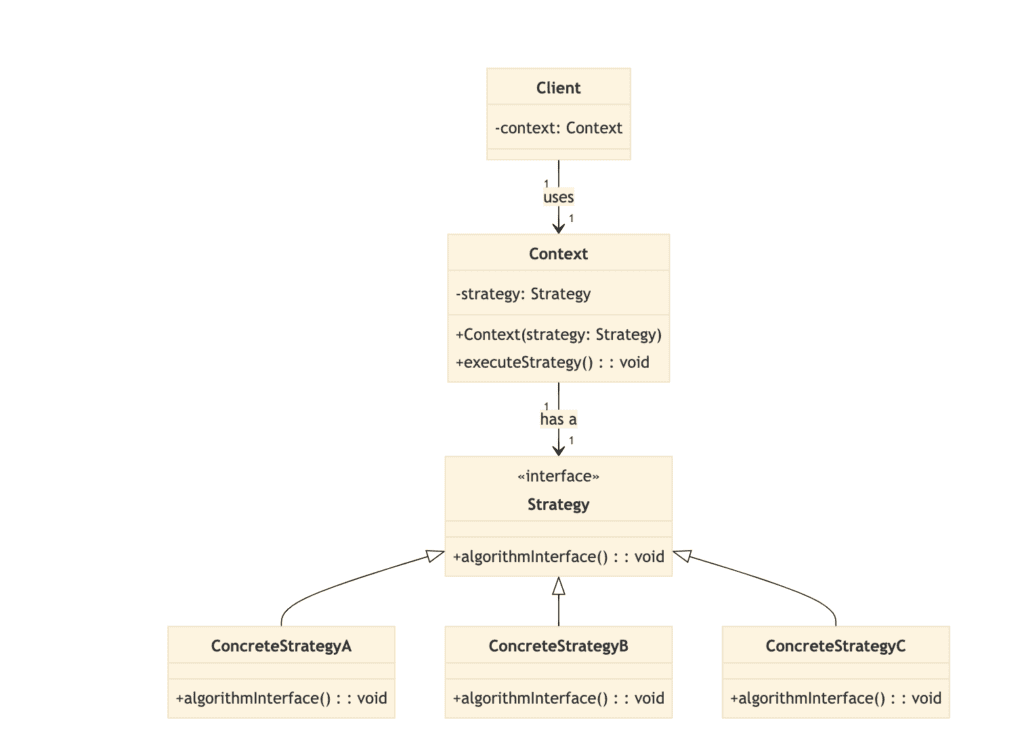
Strategy Design Pattern can be implemented by using following components:
- Strategy Interface: This defines a common interface for all supported algorithms. Each algorithm encapsulated by the strategy pattern adheres to this interface, ensuring they are interchangeable within the context where they are used.
- Concrete Strategies: These are specific implementations of the strategy interface. Each concrete strategy implements an algorithm, and the context can switch between them at runtime depending on the situation.
- Context: This is the class that contains a reference to a strategy object. The context doesn’t perform algorithmic work by itself; instead, it delegates that work to the strategy object. The context is not responsible for which algorithm is chosen; it just knows how to execute the algorithm encapsulated within the strategy.
Step-by-Step Implementation
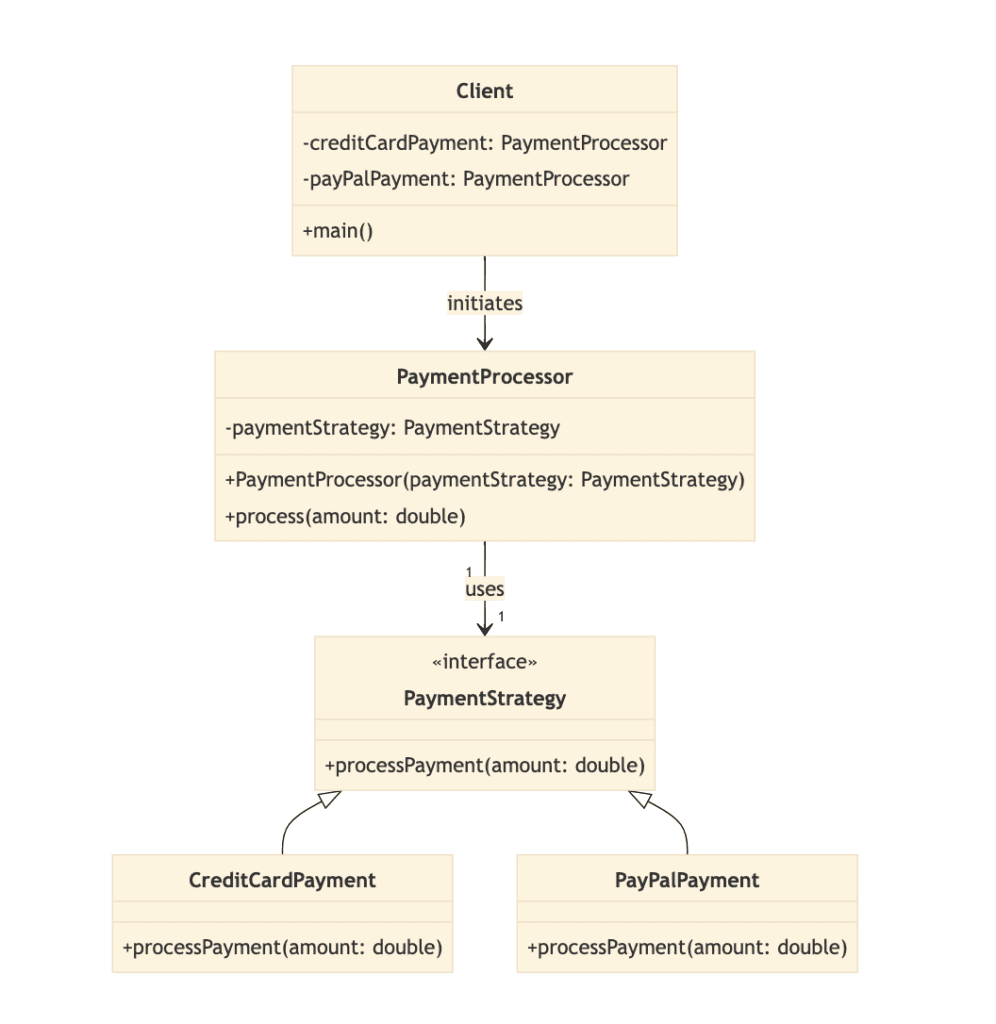
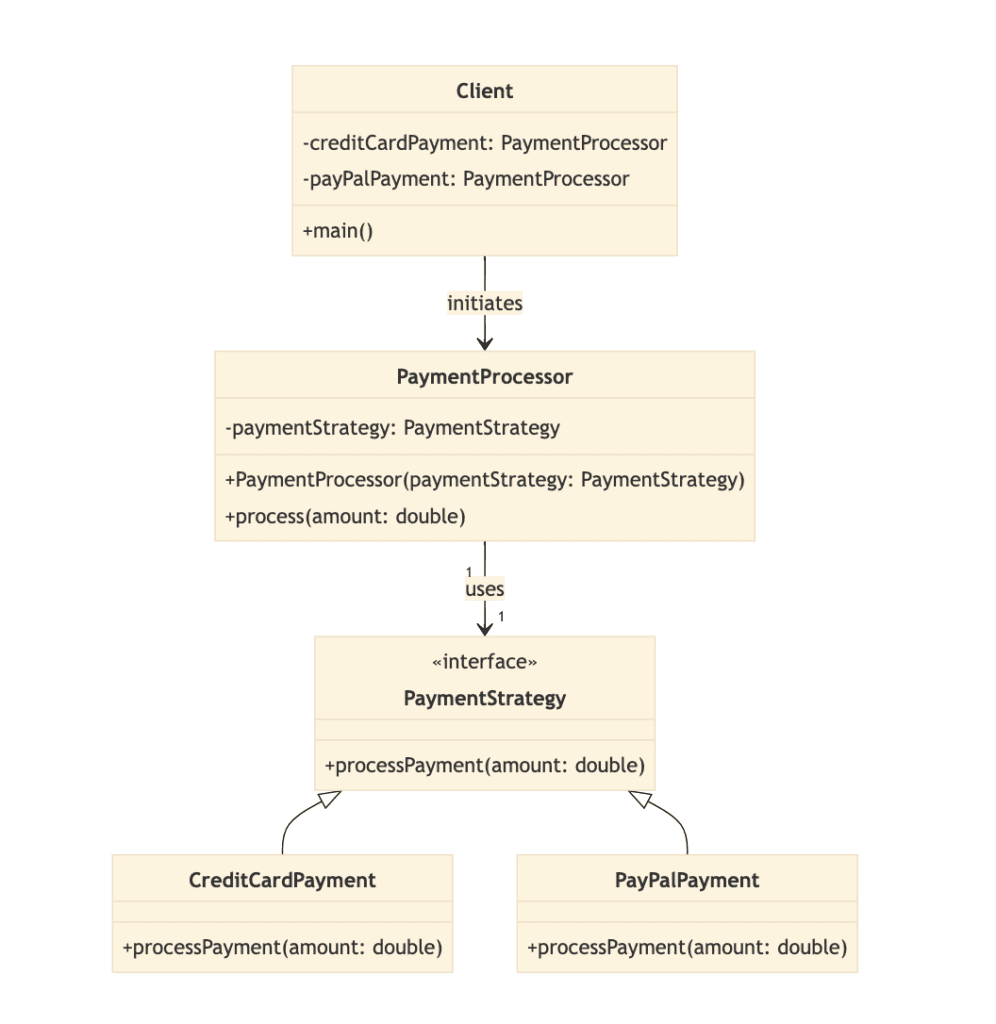
- Define the Strategy Interface:
INTERFACE PaymentStrategy
METHOD processPayment(amount: DOUBLE)
END INTERFACE
- Implement Concrete Strategies:
CLASS CreditCardPayment IMPLEMENTS PaymentStrategy
METHOD processPayment(amount: DOUBLE)
PRINT "Processing credit card payment of $" + amount
END METHOD
END CLASS
CLASS PayPalPayment IMPLEMENTS PaymentStrategy
METHOD processPayment(amount: DOUBLE)
PRINT "Processing PayPal payment of $" + amount
END METHOD
END CLASS
- Create the Context Class:
CLASS PaymentProcessor
PRIVATE paymentStrategy: PaymentStrategy
CONSTRUCTOR(PaymentProcessor, paymentStrategy: PaymentStrategy)
this.paymentStrategy = paymentStrategy
END CONSTRUCTOR
METHOD process(amount: DOUBLE)
paymentStrategy.processPayment(amount)
END METHOD
END CLASS
- Usage:
// Create a PaymentProcessor for credit card payments
creditCardPayment: PaymentProcessor = NEW PaymentProcessor(NEW CreditCardPayment())
creditCardPayment.process(100.0)
// Create a PaymentProcessor for PayPal payments
payPalPayment: PaymentProcessor = NEW PaymentProcessor(NEW PayPalPayment())
payPalPayment.process(200.0)
Now the above code by using Strategy pattern solved various issues:
- Encapsulation and Separation of Concerns: The Strategy pattern encapsulates each payment method into its own class, separating the implementation details of each strategy from the client code.
- Flexibility and Extensibility: It allows new payment methods to be added easily by creating new concrete strategy classes without modifying the existing code. We can simply create a new payment strategy class that implements the
PaymentStrategy
interface and pass it to thePaymentProcessor
. - Reduced Coupling: The client code (
Main
class) is decoupled from the implementation details of payment processing strategies. It only interacts with thePaymentProcessor
class, which takes care of invoking the appropriate strategy. This promotes code maintainability and scalability.
Common Usage of the Strategy Design Pattern
The Strategy Pattern is commonly used in:
- Sorting and Searching Algorithms: Where different algorithms might be preferred based on the context of the data.
- Payment Processing Systems: To support various payment methods without changing the system’s core logic.
- Compression Tools: Where different compression algorithms can be chosen based on user preference or data type.
- Navigational Systems: Where different routing algorithms (fastest, shortest, no-toll, etc.) can be selected dynamically.
Identifying Where To Apply Strategy Design Pattern
- Multiple Algorithms for a Task: When there are several ways to do a task, and the best method varies depending on circumstances.
- High Cohesion: When you have a class that performs a variety of actions in different ways depending on some conditions.
- **Runtime Configuration:** When the behavior of an application needs to be selected at runtime based on user input or configuration.
- Avoiding Conditional Statements: To eliminate complex conditional logic that selects between different variants of the same algorithm.
Benefits of the Strategy Design Pattern
- Flexibility: Allows for the dynamic changing of behavior within an object.
- Decoupling: Strategies and the context are decoupled, promoting loose coupling.
- Open/Closed Principle: New strategies can be introduced without changing the context, adhering to the Open/Closed Principle.
- Reusable: Strategies can be reused across different contexts.
DrawBacks of the Strategy Design Pattern
- Complexity: Introduces additional classes and interfaces, which can increase the complexity of the code.
- Clients Must Be Aware of Strategies: Clients need to know about the different strategies to choose the appropriate one.
- Increased Number of Objects: Every strategy is typically implemented as a separate object, which can lead to a proliferation of objects in the system.
Key TakeAways
- Strategy pattern decouples the implementation details of algorithms from the clients that use them. This means the client code (the context) does not need to change when a new algorithm (strategy) is added or one is modified. This separation of concerns enhances modularity and makes the system easier to understand, maintain, and extend.
- By encapsulating the algorithm behavior within separate strategy classes, the Strategy pattern allows the behavior of a class to be changed at runtime by substituting different strategy objects. This flexibility is particularly useful for applications that require dynamically changing behavior based on user input, configuration, or other conditions.
- The pattern aligns with the Open/Closed Principle, one of the SOLID principles of object-oriented design, which states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. You can introduce new strategies without altering the context or other strategies, making the system extensible.
- Each strategy can be tested independently of the context class and other strategy classes. This separation makes unit testing easier, as you can focus on testing the behavior of individual strategies without concern for their interaction with the system as a whole.
Similar Posts
What Is the Difference Between Factory and Abstract Factory Design Patterns?
When to Use Factory Design Pattern in Java?
FAQ
What is a real example of strategy design pattern?
A real-world example of the Strategy design pattern is sorting algorithms. In this scenario, the context (sorting algorithm) can utilize different strategies (e.g., bubble sort, merge sort, quick sort) interchangeably based on the user’s requirements or the characteristics of the data being sorted. By encapsulating each sorting algorithm as a strategy, the context can switch between them dynamically without modifying its code.
What is the strategy pattern in game design?
In game design, the Strategy pattern is commonly used to implement behaviors for characters or entities in the game. For example, in a strategy game, each character (e.g., warrior, mage, archer) can have different attack strategies (e.g., melee, ranged, magical) that can be selected or changed dynamically during gameplay. This allows for flexible and dynamic behavior customization without tightly coupling the behaviors to the characters.
What is difference between strategy and factory design pattern?
- The Strategy pattern is used to define a family of interchangeable algorithms, encapsulate each algorithm, and make them interchangeable. It allows the client to choose from multiple algorithms dynamically at runtime.
- On the other hand, the Factory pattern is used to create objects without specifying the exact class of object that will be created. It provides a way to delegate the instantiation of objects to subclasses, allowing the client to obtain instances of classes without knowing the specific implementation details.
What are the benefits of strategy pattern?
- Flexibility: The Strategy pattern allows algorithms or behaviors to be interchangeable at runtime, providing flexibility in choosing or switching between different strategies.
- Encapsulation: Each strategy is encapsulated within its own class, promoting code organization and modularity.
- Easy Maintenance: Adding new strategies or modifying existing ones can be done without altering the context class, leading to easier maintenance and extensibility.
- Promotes Reusability: Strategies can be reused across different contexts, promoting code reuse and reducing duplication.