Table of Contents
ToggleIntroduction
Polymorphism is among the fundamental concepts of OOP and is a term used to describe situations where something takes various roles or forms.In programming world, these things can be operator or functions. In this post we will look into various real life example of Polymorphism and also try to understand different types of polymorphism along with its implementation.
What is Polymorphism?
The term “polymorphism” is an object-oriented programming term that means ability of a function, variable or object to assume different forms. The OOO languages that exhibits polymorphism allows functions to have same name but show different behaviours within same hierarchical tree structure (i.e. classes having same parent class.)
Polymorphism is one of the important features of Object-Oriented Programming which allows us to perform a single function in different ways. In other words, polymorphism allows you to have multiple implementations under one interface. Let’s look at some of the real life example of Polymorphism.
Real Life Example Of Polymorphism
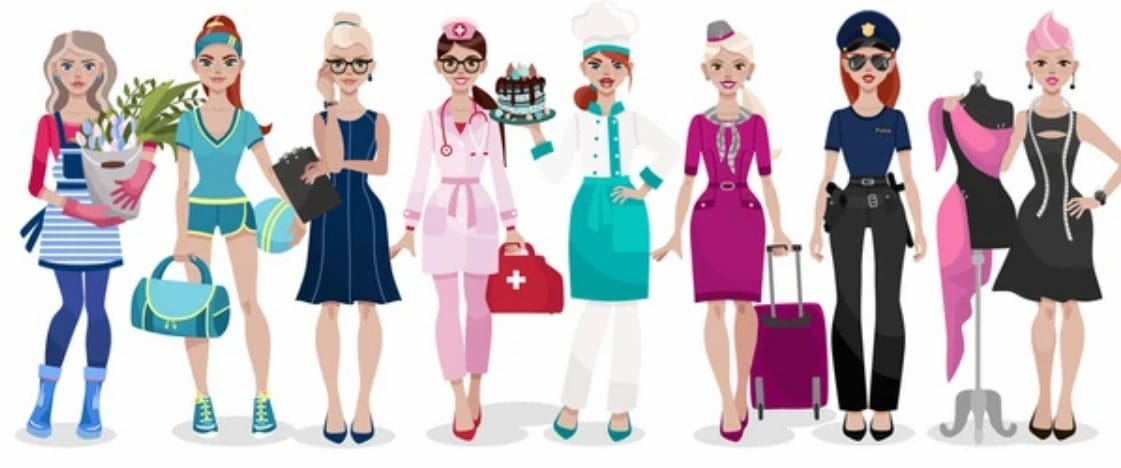
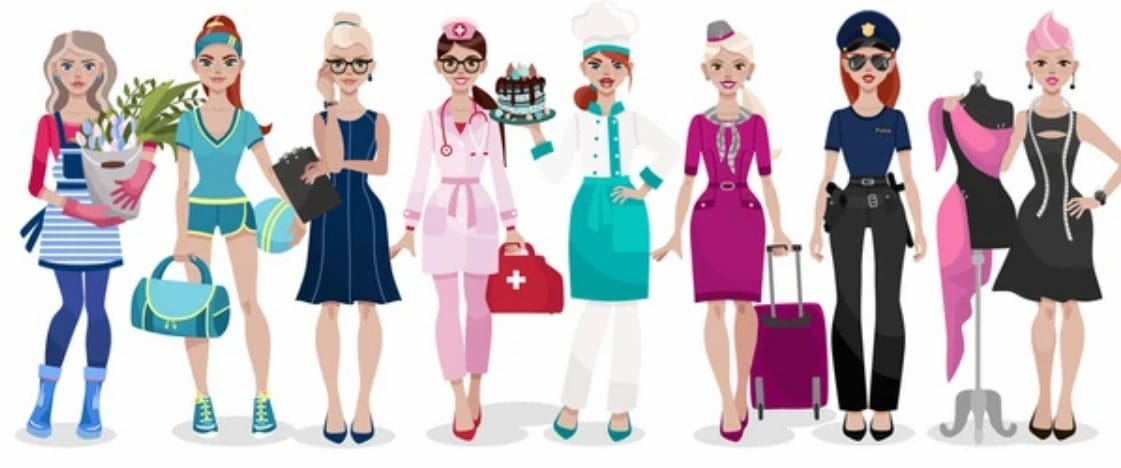
Some of the real time example of polymorphism are:
- One of the best real time example of polymorphism is Women in the society. The same woman performs different role in society.The woman can be wife of someone, mother of her child, can be at role of manager in organisation and many more at the same time. But the Woman is only one. So, same woman performing different roles is polymorphism.
- Another good real time example of polymorphism is water. Water is a liquid at normal temperature, but it can be changed to solid when it frozen, or same water changes to a gas when it is heated at its boiling point.Thus, same water exhibiting different roles is polymorphism.
- Even animals are great real life example of polymorphism, if we ask different animals to speak, they respond in their own way. Like if we ask Dog to speak it will bark, similarly, cow will moo, cat will meow. So the same action of speaking is performed in different ways by different animals exhibiting polymorphism.
- Another excellent real time example of polymorphism is your smartphone.The smartphone can act as phone, camera, music player and what not, taking different forms and hence polymorphism
- Behaviour of humans can also be considered real time example of polymorphism.Like a person behaves differently with different person.The person can be causal with his friends, professional with his colleagues and seniors. Thus Person which is object here shows different behaviour in different situation.
Types Of Polymorphism
There are two types of polymorphism
- Compile time or Static Polymorphism
- Run time Polymorphism
Compile Time Polymorphism
Polymorphism that is resolved during compiler time is known as compile time or static polymorphism.This type of polymorphism is achieved by method overloading or operator overloading.
Method Overloading: When there are multiple functions with the same name but different types or number of parameters then the functions are said to be overloaded.
// Real time example of polymorphism: Compile Time Polymorphism: Method Overloading class PerformAddition { // Method with 2 integer parameters static int add(int a, int b) { // Addition of two numbers return a + b; } // Method with same name but with different type of parameters static double add(double a, double b) { // Addition of two numbers return a + b; } // Method with same name but with different number of parameters static int add(int a, int b, int c) { // Addition of three numbers return a + b +c; } } class Main { public static void main(String[] args) { // Calling method by passing arguments System.out.println(PerformAddition.add(10,20)); System.out.println(PerformAddition.add(10.2, 20.3)); System.out.println(PerformAddition.add(10, 20, 30)); } }
In the above example which method to call is decided at compile time and it depends on type of argument passed and number of arguments passed.If no signature of arguments is matched, complier throws compile time error
Operator Overloading: In C++, operators usually work with inbuilt datatypes like int, float etc but we can make operators to work for user defined classes also. C++ has the ability to provide the operators with a special meaning for a data type, this ability is known as operator overloading. For example, Suppose we want two concat two strings in C we can overload an operator ‘+’ using operator overloading.
// Real time example of polymorphism: Compile Time Polymorphism: Operator Overloading #include <iostream> #include <string.h> using namespace std; class StringConcat { public: // character array for storing string char str[20]; StringConcat() {} StringConcat(char str[]) { strcpy(this->str, str); } // Operator Overloading + for string concatenation StringConcat operator + (StringConcat& obj2) { StringConcat obj3; // strcat() to concat two specified string strcat(this->str, obj2.str); // Copy the string to string to be return strcpy(obj3.str, this->str); return obj3; } }; int main() { char str1[] = "I Love"; char str2[] = "Programming"; StringConcat obj1(str1); StringConcat obj2(str2); // Call the operator function StringConcat obj3 = obj1 + obj2; cout << "String Concatenation Using Operator Overloading: " << obj3.str; return 0; } int main() { char str1[] = "I Love"; char str2[] = "Programming"; StringConcat obj1(str1); StringConcat obj2(str2); // Call the operator function StringConcat obj3 = obj1 + obj2; cout << "Concatenation: " << obj3.str; return 0; }
Runtime Polymorphism
The polymorphism which gets resolve dynamically at the runtime rather than compile-time is called runtime or dynamic polymorphism. It is also called dynamic binding or Dynamic Method Dispatch.Run time polymorphism can be achieved using Method Overriding.
Method Overriding occurs when a derived class also has a definition for one of the member functions of the base class and therefore base function is said to be overridden. The call to function is resolved by the of object calling the function.
// Real life example of polymorphism: Run time Polymorphism class Base { // Method of base class void print() { System.out.println("In base class print function"); } } // Child class 1 class Child1 extends Base { // Method overriden void print() { System.out.println("In child1 class print function"); } } // Child class 2 class Child2 extends Base { // Method overriden void print() { System.out.println("In child2 class print function"); } } public class Main { public static void main(String[] args) { //Base Cass object Base b = new Base(); b.print(); b = new Child1(); b.print(); b = new Child2(); b.print(); } }
In the above java program, which function to call that is resolved at run time dynamically.
Conclusion
In this article we covered polymorphism and its types, we also looked into some real world example of polymorphism.In summary, ability of same object or function to perform different roles is called polymorphism.
Got a question or just want to chat? Comment below or drop by our forums, where a bunch of the friendliest people you’ll ever run into will be happy to help you out.