Table of Contents
ToggleThread priority In Java is an important concept in Java that allows developers to provide hints to the thread scheduler about the relative importance of different threads.
What is Thread Priority In Java?
In simple terms, thread priority in java refers to assigning priorities to different threads to indicate which Thread should execute first and which can wait. The scheduler uses these priorities to decide the order in which threads are executed.
By default in Java, every Thread is given a priority inherited from the Thread that created it. The main Thread, which begins execution, is given the default priority of the Thread.NORM_PRIORITY, which is 5. Subsequently, any threads spawned by the main Thread will get the same default priority.
However, the priority of individual threads can be changed based on their importance.
- Thread priorities in Java range between:
- 1 (Thread.MIN_PRIORITY)
- 10 (Thread.MAX_PRIORITY)
- The higher the numerical value, the higher the Thread’s priority.
Setting the right priority for the right tasks allows developers to improve the performance and responsiveness of their applications. In this article, we will look into details on how to set and get thread priority in Java. So, let’s dive into it.
How to set Priority of Thread in Java?
The priority level of a thread can be dynamically adjusted using the setPriority() method in java, a feature of the Java Thread class. This functionality allows for more refined control over the execution of concurrent processes.
Thread Priority Levels In Java
Java defines three standard priority levels for threads:
- MIN_PRIORITY (1): Represents the lowest possible priority for a thread. Ideal for background or less critical tasks.
- NORM_PRIORITY (5): The default priority level for a thread and generally suitable for most applications.
- MAX_PRIORITY (10): The maximum thread priority in java for tasks requiring immediate attention.
These priority levels are implemented as public static final constants within the Thread class, ensuring easy access and modification.
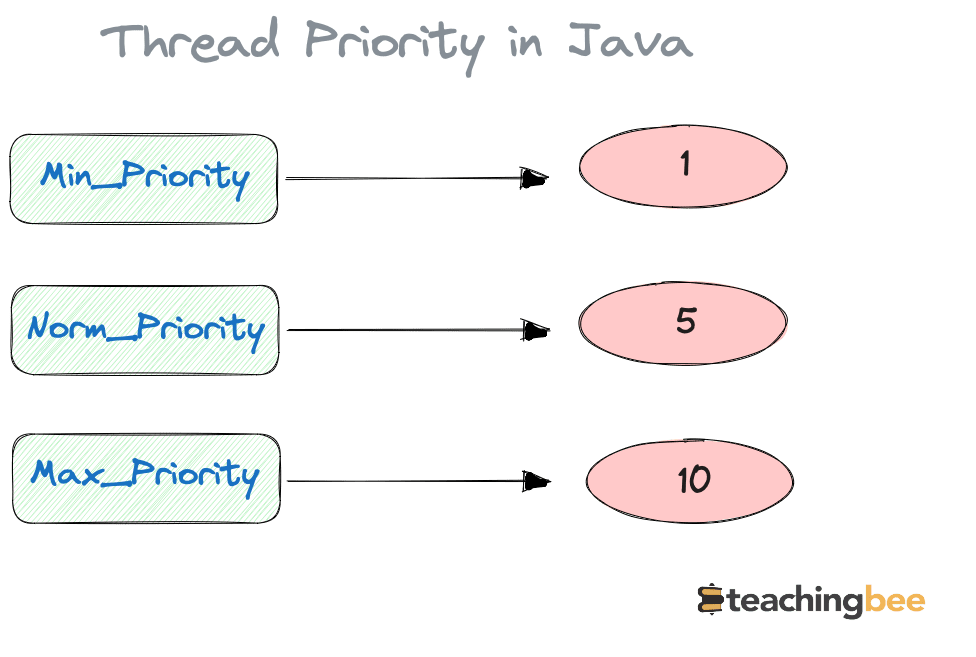
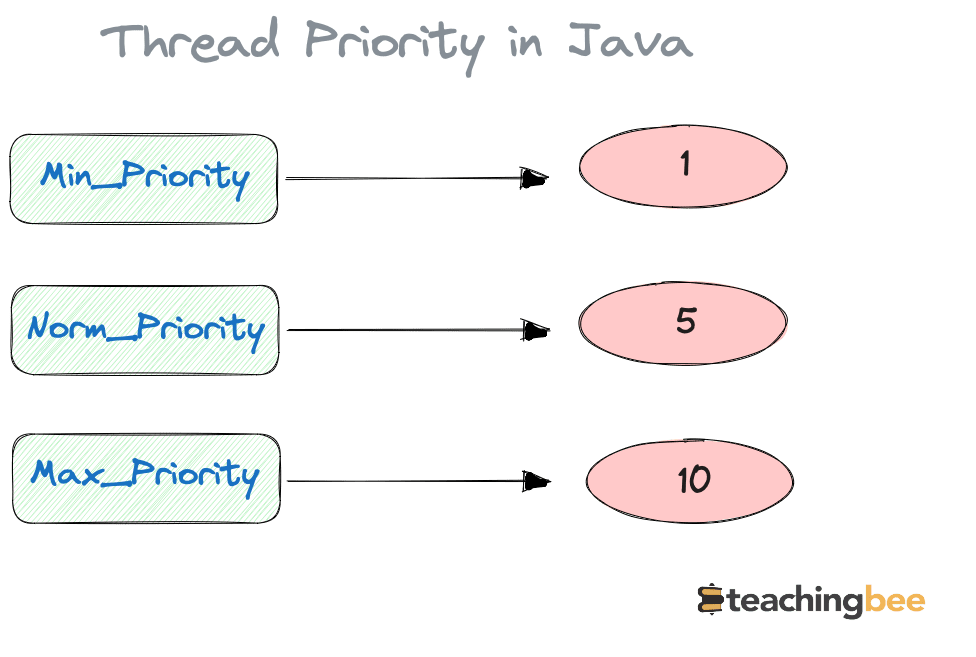
Using setPriority() Method In Java
The setPriority() method adjusts a thread’s priority and accepts an integer parameter ranging from 1 (MIN_PRIORITY) to 10 (MAX_PRIORITY).
Here’s an example demonstrating its usage:
// Set Priority Of Thread In Java
Thread t = new Thread();
t.setPriority(Thread.MAX_PRIORITY); // Sets thread 't' to maximum priority
In this code snippet, the Thread’s priority is set to the highest level, 10. A higher priority suggests that thread t will have a higher chance of execution than other threads with lower priorities.
Example of Priority of a Thread in Java
import java.lang.*;
public class JavaThreadPriorityExample extends Thread {
public void run() {
// Output to indicate the run() method execution
System.out.println("Running in the run() method");
}
public static void main(String argvs[]) {
// Creating threads
JavaThreadPriorityExample thr1 = new JavaThreadPriorityExample();
JavaThreadPriorityExample thr2 = new JavaThreadPriorityExample();
JavaThreadPriorityExample thr3 = new JavaThreadPriorityExample();
// Displaying default priorities
System.out.println("Thread Priority of thr1: " + thr1.getPriority());
System.out.println("Thread Priority of thr2: " + thr2.getPriority());
System.out.println("Thread Priority of thr3: " + thr3.getPriority());
// Setting new priorities
thr1.setPriority(7);
thr2.setPriority(4);
thr3.setPriority(9);
// Displaying new priorities
System.out.println("New Thread Priority of thr1: " + thr1.getPriority());
System.out.println("New Thread Priority of thr2: " + thr2.getPriority());
System.out.println("New Thread Priority of thr3: " + thr3.getPriority());
// Main thread details
System.out.println("Current Running Thread: " + Thread.currentThread().getName());
System.out.println("Main thread Priority: " + Thread.currentThread().getPriority());
// Setting priority of the main thread
Thread.currentThread().setPriority(10);
System.out.println("New Main thread Priority: " + Thread.currentThread().getPriority());
}
}
Output
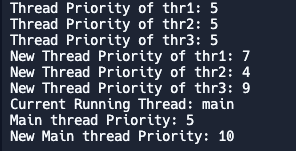
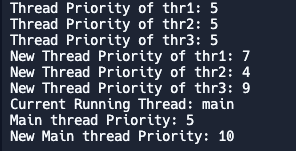
How to get Priority of Thread in Java?
In Java, monitoring the priority of threads is essential for effective thread management, especially in complex applications. The getPriority() method is a key tool in this process, enabling developers to retrieve the current priority of a thread.
Using getPriority() Method In Java
- The getPriority() method is an instance method within the Thread class. It returns the current priority of the Thread as an integer value.
- This method is particularly useful for verifying the priority level at runtime, which can be crucial for debugging and performance tuning.
Consider the following example, which demonstrates the usage of getPriority():
// Getting Thread Priority In Java
Thread t = new Thread();
t.setPriority(Thread.MAX_PRIORITY); // Setting the thread's priority to maximum
int priority = t.getPriority(); // Retrieving the current priority
System.out.println("Priority: " + priority); // Output: Priority: 10
In this snippet:
- A new thread t is created, and its priority is set to MAX_PRIORITY (10).
- The getPriority() method is invoked on t to obtain its current priority.
- The retrieved priority (10) is then printed, confirming the Thread is set at the maximum level.
Default Thread Priority in Java
Java provides default priority of threads when new thread is created.
Default Priority Assignment
- Initial Main Thread Priority: When a Java application starts, the main() method initiates the main Thread. This Thread is automatically assigned a default priority, typically NORM_PRIORITY, which is numerically represented as 5.
- Inheritance of Priority: Threads spawned from the main Thread, or any other thread, inherit the priority of their parent thread. This inheritance principle is crucial in maintaining a consistent and predictable threading environment.
// Default thread priority in java
public class Main {
public static void main(String[] args) {
Thread t1 = new Thread(); // Inherits default priority from the main thread
Thread t2 = new Thread(); // Similarly, inherits default priority
// Both t1 and t2 will have a priority of 5 (NORM_PRIORITY)
}
}
In this example:
- t1 and t2 are new threads created within the main method.
- Both t1 and t2 inherit the default priority of the main Thread, which is NORM_PRIORITY or 5.
Why Is Thread Priority In Java Important?
The priority value assigned to a thread in Java is a hint or guidance for the thread scheduler to determine which Thread should execute first. The general rule is that threads with a higher priority value will be scheduled to run by the thread scheduler before threads with a lower priority value.
For example, suppose there are three threads – A, B, and C with priorities 8, 5, and 3, respectively. When these threads compete for CPU time, the thread scheduler will typically execute Thread A first, followed by Thread B, and then Thread C. The priority value indicates the intended order of execution.
However, it is important to note that thread priorities do not guarantee that order will always be maintained. The thread scheduler may sometimes override the priority ordering based on other factors like:
- Synchronization locks – A lower-priority thread may already hold a lock needed by a higher-priority thread. In that case, the lower priority thread will continue execution until it releases the lock.
- I/O blocking – A higher priority thread may get blocked on I/O, allowing lower priority threads to execute.
- Parallelism – Two threads of different priorities may execute in parallel on multi-core systems.
- OS scheduling – The operating system’s scheduling algorithms also influence scheduling.
In summary, thread priorities guide the scheduler but do not strictly define the execution order. The scheduler may change the order based on synchronization, available cores, I/O blocking, and other factors. Developers should be aware that priorities do not guarantee ordering.
Best Practices For Thread Priority In Java
When working with thread priorities in Java, there are some best practices and guidelines developers should follow:
- The main Thread should normally have the default priority (NORM_PRIORITY or 5). Increasing the priority of the main Thread can lead to the starvation of other threads.
- Threads handling critical tasks like updating the UI, processing user inputs, etc., should have a slightly higher priority. This improves responsiveness and performance.
- Avoid setting very low priorities like MIN_PRIORITY unless there is a specific requirement. Very low-priority threads may need more CPU time, leading to starvation.
- In general, use NORM_PRIORITY for most threads. Only tweak priorities when there is a performance issue and a need to prioritize some threads.
- Change thread priorities carefully and one at a time to assess impacts. Drastic priority changes can negatively impact performance instead of improving it.
- Make priority changes consistently across related threads. For example, increase priority for all UI handling threads rather than just one.
- Document priority changes made in the code using comments so other developers know it.
- Monitor CPU usage and thread activity after priority changes to verify improvements. Profile carefully.
Potential Issues While Handling Thread Priority In Java
While thread priorities provide a useful mechanism to influence scheduling, improper usage can also lead to some common concurrency issues:
Race Conditions
- If shared data accessed by multiple threads is not synchronized properly, changing priorities can cause race conditions.
- A higher-priority thread may access stale data before a lower-priority thread updates it.
- To avoid this, use synchronized blocks or other locking to ensure access is sequentialized.
Deadlock
- Inappropriately synchronizing code can lead to threads waiting indefinitely, causing deadlock.
- A classic example is a high-priority thread holding a lock that a lower-priority thread needs.
- Carefully structure locks and avoid nested/circular locks.
- An unfavorable priority configuration can cause threads to get stuck, retrying an operation.
- But no thread ever makes progress, leading to a livelock situation.
- Tuning thread priorities and avoiding unnecessary retries can prevent livelocks.
The key is to analyze performance bottlenecks carefully and change thread priorities gradually. Rushing to make significant priority changes without proper testing often compounds problems.
Key Takeaways
- Thread priority hints to the scheduler and does not strictly define execution order. The scheduler can override priorities.
- Default thread priority is inherited from the parent thread, usually NORM_PRIORITY (5) for the main Thread.
- Use setPriority() to change thread priorities when tuning performance. But change carefully.
- Follow best practices like avoiding high or low priorities to prevent issues.
- Monitor CPU usage and thread activity when adjusting priorities to verify improvements. Look for race conditions, deadlocks, or livelocks.
Checkout more Java Tutorials here.
I hope You liked the post ?. For more such posts, ? subscribe to our newsletter. Try out our free resume checker service where our Industry Experts will help you by providing resume score based on the key criteria that recruiters and hiring managers are looking for.
FAQ
Why do we need thread priority in Java?
Thread priority in Java helps determine the order in which threads are scheduled for execution. It allows for more efficient use of CPU resources by enabling prioritization of more critical tasks, thereby improving application performance and responsiveness.
What happens if two threads have the same priority in Java?
When two threads have the same priority in Java, the thread scheduler decides their execution order based on thread scheduling policies, which can be either time-slicing or preemptive. In such cases, the execution order is less predictable and may depend on the thread scheduler’s implementation in the Java Virtual Machine (JVM) and the underlying operating system.
Can we change the priority of the main Thread in Java?
Yes, we can change the priority of the main Thread in Java. This is done by calling the setPriority() method on the main Thread, just like with any other thread. However, it’s important to do this judiciously, as changing the main Thread’s priority can affect the overall application’s behaviour and performance.
What Is Maximum Thread Priority In Java
The maximum thread priority in Java is defined by the constant Thread.MAX_PRIORITY
, which has a value of 10. This is the highest priority that can be assigned to a thread in Java, indicating that the thread should be given preference in scheduling over threads with lower priorities.