Table of Contents
ToggleIntroduction
For any programmer, arrays are as vital as air. These simple, powerful data structures exist at the core of almost every non-trivial program or algorithm. From the mighty databases that run the internet to the humblest app on your smartphone, arrays provide the skeletal framework on which everything is built.
By the end of this guide, you’ll have an intimate grasp of what makes arrays tick – their syntax, properties, uses, advantages and disadvantages of array. With vivid examples, you’ll gain not just technical knowledge, but an intuitive feel for how arrays enable efficient coding.
So join us on a richly illustrated tour through the world of arrays! Regardless of whether you’re a newbie or seasoned pro, by the end you’ll gain a deeper mastery of these essential data structures that form the backbone of computer programming.
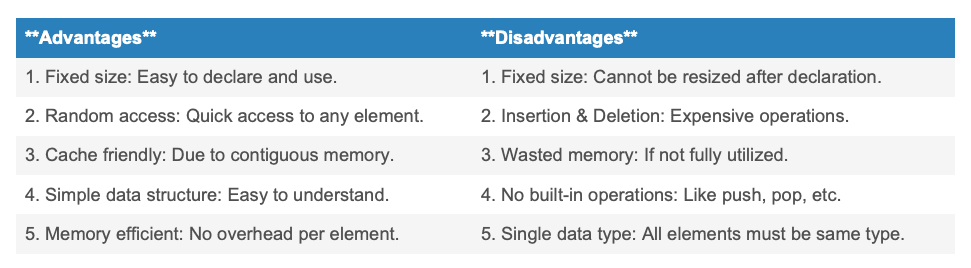
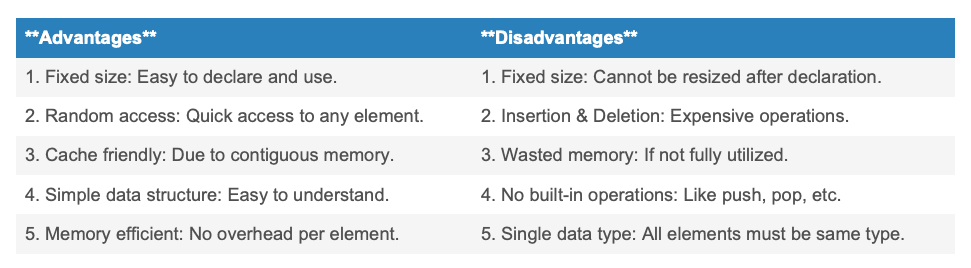
Advantages And Disadvantages of Array
Let’s look into some of advantages and disadvantages of array.
Advantages of Arrays
Arrays are one of the most commonly used data structures in programming. They offer several benefits that make them indispensable for organizing and storing data efficiently. Let’s examine some of the key advantages of using arrays.
Random Access
Arrays allow random access to elements using indices – this means you can directly access any element without having to iterate through the elements sequentially.
For example:
// Advantages And Disadvantages of Array: Random Access in Array int[] arr = {1, 2, 3, 4, 5}; int thirdElement = arr[2]; // directly access third element
This provides performance benefits as you can reach any index position in constant O(1) time. Arrays are optimal when you need frequent indexed access.
Ease of Traversal
Traversing array elements sequentially is very easy using a loop. You can iterate through the entire array to process all elements.
// Advantages And Disadvantages of Array: Easy Traversal of Arrays using loops for (int i = 0; i < arr.length; i++) { // process element arr[i] }
This simplicity makes arrays ideal for sequential access in iterative algorithms.
Memory Allocation
Arrays allow contiguous allocation of memory for all elements. The entire block of memory allocated can be accessed directly using pointers.
This makes lookup very fast as the memory is cached efficiently by leveraging locality of reference. It also minimizes fragmentation issues during allocation.
Use in Multi-dimensional Structures
Arrays can be used to construct multi-dimensional data structures like matrices and tensors which are often used in scientific computations and machine learning.
For example, a matrix can be constructed as a 2D array of arrays in Python:
// Advantages And Disadvantages of Array: Multi-dimensional Structure matrix = [[1, 2, 3], [4, 5, 6]]
The nested array structure allows efficient storage and access of multi-dimensional data.
Performance
Arrays allow fast iterations and batch operations using index mapping. This enables optimized bulk processing.
For example, multiplying each element in an array by a factor x can be done simply:
// Advantages And Disadvantages of Array: Array Performance for(int i = 0; i < arr.length; i++){ arr[i] *= x; }
This loop executes in O(n) time. Arrays enable such fast batch computations crucial for performance.
Advantages | Description |
---|---|
Random Access | Directly access any element without iterating through the elements sequentially. O(1) time for indexed access. |
Ease of Traversal | Arrays can be easily traversed sequentially using loops, making them ideal for iterative algorithms. |
Memory Allocation | Contiguous allocation of memory for all elements, leveraging locality of reference and minimizing fragmentation. |
Use in Multi-dimensional | Arrays can be used to construct multi-dimensional structures like matrices and tensors. |
Structures | |
Performance | Fast iterations and batch operations using index mapping enable optimized bulk processing. |
In summary, arrays provide fast access, ease of traversal, efficient memory usage, multi-dimensional modeling and performance benefits making them indispensable in most programs. Their simplicity and versatility cement their place as one of the most widely used data structures.
Disadvantages of Array
While arrays are ubiquitous and offer many advantages, they also come with some limitations. Let’s look at some key disadvantages of using arrays.
Fixed Size Limitation
The size of arrays is fixed when they are created. This can be problematic when working with dynamic data:
// Disadvantage of Array: Fixed Size int[] arr = new int[5]; //can only hold 5 elements
If you exceed the capacity, you need to create a new larger array and copy elements over which is expensive. Some languages offer dynamic arrays that can resize, but those come with overheads too.
This makes arrays rigid for use cases where data size is unpredictable or grows/shrinks frequently.
Insertion and Deletion
Inserting and deleting elements in arrays is expensive compared to data structures like linked lists.
To insert at index i:
// Disadvantage of Array: Insertion and Deletion //shift elements i onwards one position ahead for(int j=size; j>i; j--){ arr[j] = arr[j-1]; } arr[i] = value;
This takes O(n) time since shifting requires moving n elements. Deletion follows a similar process of shifting elements after removal.
Memory Wastage
Arrays can waste memory if they are not fully populated. The allocated memory cannot be de-allocated if array size reduces:
// Disadvantage of Array: Memory Wastage int[] arr = new int[1000]; //allocates memory for 1000 elements arr.length = 100; //only using 100 elements but 1000 allocated
The unused memory remains allocated leading to wastage.
No Special Operations
Unlike other data structures like Stack, Queue, Tree etc., arrays do not provide special operations like peek, push, traverse etc. You only get basic insertion, deletion and access.
They also lack features like ordering and searching that more complex data structures provide. Arrays contain just raw data without intelligent organization.
Disadvantages | Description |
---|---|
Fixed Size Limitation | The size of arrays is fixed when created. If data grows, a new larger array is needed, leading to overhead. |
Insertion and Deletion | Inserting and deleting elements is expensive due to the need to shift other elements. |
Memory Wastage | If arrays are not fully populated, they can waste memory. Memory remains allocated even if not used. |
No Special Operations | Arrays lack special operations like peek, push, traverse etc. They also lack features like ordering and searching. |
In summary, arrays have fixed sizes, slow inserts and deletes, potential memory wastage, and lack of built-in operations. However, with some design forethought, these limitations can be mitigated to use arrays effectively. The pros of arrays usually outweigh the cons for most applications.
Comparative Analysis of Advantages and Disadvantages of Array
Arrays vs. Linked Lists
Here is a comparison of arrays and linked lists in a tabular format:
Feature | Array | Linked List |
---|---|---|
Declaration | DataType[] arr; | LinkedList list; |
Memory Allocation | Contiguous | Non-contiguous |
Size | Fixed | Dynamic |
Access Time | O(1) – Direct index access | O(n) – Sequential access |
Insertion Time | O(n) – Shifting required | O(1) – Change pointers |
Deletion Time | O(n) – Shifting required | O(1) – Change pointers |
Common Operations | get(), set(), traverse() | insertFirst(), removeLast(), search() |
Use Cases | Storing and accessing sequential data, matrices | Implementing queues, stacks, graphs |
Advantages | Fast access, memory locality | Dynamic size, efficient inserts/deletes |
Disadvantages | Fixed size, expensive inserts/deletes | Slow sequential access |
Key Differences:
- Arrays have fixed sizes and allow fast O(1) index-based access. Linked lists have dynamic sizes and require sequential O(n) access.
- Insertions and deletions are faster in linked lists since pointers can be directly changed. Arrays require shifting elements which is O(n).
- Arrays are better for accessing and manipulating data sets. Linked lists suit applications with frequent changes like queues and graphs.
- Memory is allocated contiguously for arrays while linked lists can fragment memory by allocating non-contiguous nodes.
Learn about difference between queue and other data structure .
Conclusion
Arrays are one of the most fundamental data structures used in programming. Their simplicity yet versatility has cemented their place at the core of countless algorithms and applications. When discussing the advantages and disadvantages of array, one of the significant advantages is providing fast O(1) indexed access to elements. This random access enables efficient reading and manipulation of data sets. Traversal is also straightforward with simple iterative loops. Arrays allow contiguous allocation of memory, leading to better caching and locality of reference. Multi-dimensional data can be modeled intuitively using nested arrays.
However, the disadvantages of arrays include having a fixed size that requires preallocation and cannot dynamically adjust. Insertions and deletions are expensive as elements need to be shifted. There is potential for wasted unused memory if arrays are sparsely populated. They also lack built-in methods for common data structure operations like sorting, stacks, etc.
Learning to leverage arrays effectively is key to enhancing your programming skills. They offer a solid foundation to build adaptable and optimized solutions. A deeper understanding of arrays can elevate your general comprehension of computer science concepts and data-oriented thinking.
Try out our free resume checker service where our Industry Experts will help you by providing resume score based on the key criteria that recruiters and hiring managers are looking for.
Frequently Asked Questions (FAQs)
Q: When should I use arrays vs lists?
A: Use arrays if you need indexed/random access. Use lists if you need to frequently add/remove elements.
Q: Can array size change dynamically in languages like Java?
A: No, array size is fixed in Java after declaration. But some languages like Python offer dynamic arrays that can resize.
Q: Is inserting into array O(1) or O(n)?
A: Insertion in arrays is O(n) since elements may need to be shifted. Linked lists allow O(1) insertion.
Q: How do deletions work in arrays?
A: Deletion is O(n) as elements are shifted to fill the index gap. Lists allow faster O(1) deletion.
Q: Are multidimensional arrays possible?
A: Yes, arrays can be nested to create 2D and higher multidimensional arrays like matrices.
Q: What are associative arrays?
A: Associative arrays or maps allow indexing via key-value pairs instead of just sequence-based indices.
Q: How are sparse arrays different from normal arrays?
A: Sparse arrays optimize storage for arrays where most elements are zero or empty. Normal arrays store zeroes as well.
Useful Resources
- GeeksforGeeks Arrays Section – Great reference for array syntax, operations and questions.
- Visualgo Array Visualizations – Interactive visualizations of array operations and algorithms.
- CS50 Array Lectures – Video lectures explaining arrays from basics to applications.
- Real Python Arrays Article – Array types, operations, numpy arrays with Python examples.