Table of Contents
ToggleIntroduction
This article will provide a comprehensive overview of compilation in Java with example. We’ll start with the basics of compilation in java and the role of the Java compiler. Then we’ll explore the key differences between compilation and execution in Java.
You’ll learn the step-by-step Java compilation process, how the compiler works, and the role of the JVM. We’ll also cover essential syntax for avoiding errors, advanced compilation techniques, best practices, and troubleshooting advice.
By the end, you’ll have a solid grasp of compilation in java to confidently write and deploy your code.
Importance of Compilation in Java Programming
Java is a compiled language which means code must be compiled before it can be executed. Understanding compilation is critical for optimising code performance, building efficient applications, and debugging errors. Mastering Java compilation makes development work seamlessly.
The Basics of Compilation in Java
What is Compilation?
Compilation is the process of translating Java source code into bytecode that can then be executed by the JVM (Java Virtual Machine). The Java compiler handles this translation.
Source code is written in high-level Java programming language. The compiler converts it into low-level byte code instructions that the JVM understands.
The Role of the Java Compiler
The Java compiler (javac) analyzes source code for errors and converts valid code into bytecode (.class files). It ensures code adheres to Java language rules and syntax.
The compiler optimizes performance by translating high-level code to more efficient bytecodes. It also catches errors early in the development process.
Compilation in Java vs Execution
Understanding the Difference
In Java, compilation and execution are distinct sequential steps:
- Compilation – Source code is compiled into bytecode
- Execution – Bytecode is interpreted and executed by the JVM
Compilation happens once, while execution can happen multiple times. Compilation checks syntax and converts code. Execution actually runs the program logic.
How Java Code Transforms into Bytecode
Java source code (.java files) containing classes and methods get compiled into .class files that contain Java bytecode.
This bytecode gets interpreted by the JVM at runtime to actually execute the program. So compilation converts code into an executable form.
Compilation in Java with Example
Pre-compilation Steps
Before starting compilation, ensure:
- Source code is stored in .java files
- Code is organized into appropriate classes and methods
- Required classes and packages are imported
- Code follows Java syntax rules
Invoking the Java Compiler
Compiling Java code involves:
- Invoking the javac compiler
- Compiler parses source code
- Checking for syntax errors
- Type checking source code
- Generating bytecode if no errors found
- Outputting .class bytecode files
Once the source code is ready, it’s time to compile it. This is done using the javac
command followed by the filename. For example: javac MyProgram.java
Generation of Bytecode
The javac
compiler reads the .java
file and translates it into bytecode, saving it with a .class
extension. This bytecode is platform-independent, meaning it can run on any device with a JVM.
Post-compilation Steps
After successful compilation:
- Bytecode can be packaged into a JAR file
- JARs and class files can be executed by JVM
- Code may require additional runtime resources
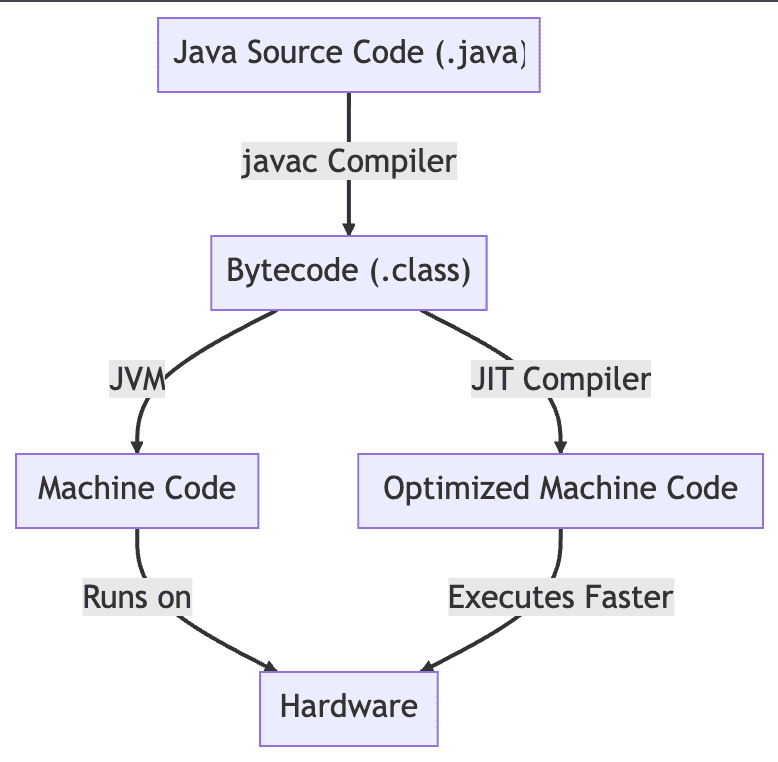
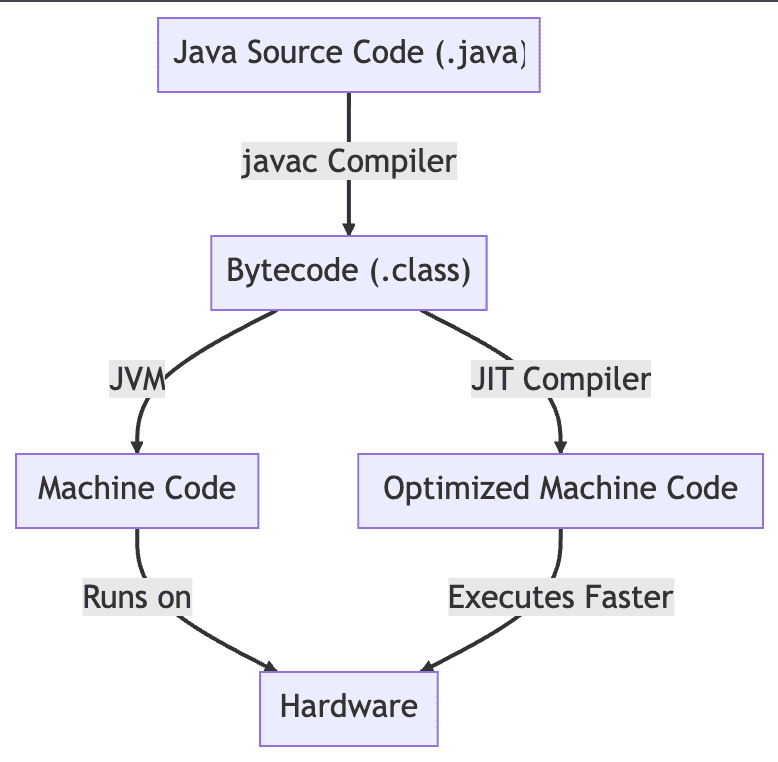
Compilation in Java Example
Let’s consider a simple “Hello World” program to understand the compilation process better.
// public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } } //
After writing the above code in a file named HelloWorld.java
, you would compile it using: javac HelloWorld.java
. This command will produce a HelloWorld.class
file containing the bytecode.
Deep Dive into Java Compiler
Types of Java Compilers
There are different implementations of the javac compiler:
- Oracle’s javac – Default compiler included in JDK
- Eclipse Compiler for Java (ECJ) – Used by Eclipse IDE
- GraalVM Compiler – Polyglot high-performance compiler
How Does the Java Compiler Work?
The javac compiler’s stages include:
- Lexical analysis – Breaks input into tokens
- Syntax analysis – Confirms syntax is valid
- Semantic analysis – Contextual analysis for meaning
- Intermediate code generation – Platform-independent bytecode
- Code optimization – Improves code efficiency
Error checking occurs at each stage.
Java Virtual Machine (JVM) and Compilation
What is JVM?
The JVM is a virtual machine that executes Java bytecode. It provides a runtime environment and is responsible for executing the compiled code.
Tiered Compilation in JVM
The JVM uses tiered compilation for improved performance:
- Interpreter – Quickly interprets simple bytecode
- JIT compiler – Compiles bytecode during execution for efficiency
- Garbage Collector – Removes unused objects
Syntax Essentials for Java Compilation
Importance of Syntax in Compilation
Java’s syntax rules dictate how code must be structured for successful compilation. Key elements include:
- Proper statements termination with semicolons
- Correct use of brackets in blocks and methods
- Proper variable declarations and initialization
- Valid method overloading/inheritance syntax
Common Syntax Errors to Avoid
Some syntax errors that can prevent compilation:
- Missing semicolons, brackets, parentheses
- Unclosed strings and code blocks
- Invalid variable, method, class names
- Type mismatch errors
- Access and scope errors
Careful coding adhering to syntax helps avoid these errors.
Advanced Topics in Java Compilation
Just-In-Time Compilation
While the bytecode is platform-independent, it still needs to be translated into machine code for execution. This is where the JVM and JIT compiler come into play. JIT compilation compiles bytecode during runtime for boosted performance. The JIT compiler selectively compiles hot spots that benefit most from native code.
Advantages of JIT Compilation
- Performance Boost: By compiling only the necessary parts of bytecode, execution becomes faster.
- Optimization: The JIT compiler can apply various optimizations during runtime, enhancing performance.
- Memory Efficiency: Since only parts of the bytecode are compiled, less memory is used.
Ahead-Of-Time Compilation
AOT compilation converts bytecode to native machine code during build time rather than runtime. This increases startup speed since code doesn’t need compilation.
Best Practices for Java Compilation
Code Optimization Tips
- Use final variables where possible
- Declare methods and variables with smallest scope
- Enable JIT compiler optimizations
- Use profiling to identify hot spots
Debugging and Troubleshooting
- Read compiler error messages carefully
- Use IDE debugging features and breakpoints
- Consult stack traces for clues
- Refactor complex code and minimize dependencies
- Check third party libraries for issues
Conclusion
Summary of Key Points
We covered a complete overview of Java compilation:
- The compile-time translation of code to bytecode
- Distinctions between compilation vs execution
- Step-by-step guide to the compilation process
- How JIT and AOT compilation work
- Essential syntax and best practices
Final Thoughts on Mastering Compilation in Java
Understanding compilation helps write optimized Java code. Learning its intricacies will help you become a skilled Java developer.
With the knowledge from this article, you can confidently tackle compilation tasks and get the most out of your Java applications.
FAQ Related To Compilation In Java
What are the 2 steps in Java compilation?
The two main steps in Java compilation are:
- Compilation – converts Java source code to bytecode
- Execution -runs the bytecode to generate the program output
The two high-level steps are:
- javac compiles .java -> .class
- JVM executes .class -> output
The compilation step converts Java source code to bytecode, while the execution step runs the bytecode to generate the program output.
What are the 5 phases of Java compilation?
The 5 phases of Java compilation are:
- Parsing
- Syntax Analysis
- Semantic Analysis
- Intermediate Code Generation
- Code Optimization
What is compilation and interpretation in Java?
Java is a compiled language whereas Python is an interpreted language. In compilation, the code is converted to platform-independent bytecode by the Java compiler at build time before execution. This improves performance. In interpretation, the Python interpreter executes the code line-by-line during runtime. This allows for dynamic behavior but is slower. Java’s ahead-of-time compilation enables improved optimization, security, portability and faster execution compared to Python’s runtime interpretation. However, Python’s interpretation allows for more flexibility and iteration during development. Ultimately, compilation trades runtime flexibility for better performance, while interpretation provides dynamism at the cost of speed.
What is JVM JRE and JDK?
Here are one line descriptions of JVM, JRE, and JDK:
JVM – The Java Virtual Machine that executes Java bytecode.
JRE – The Java Runtime Environment that provides the minimum requirements for running Java code.
JDK – The Java Development Kit that provides tools including the Java compiler and JRE needed to develop Java applications.
Additional Resources
- Effective Java by Joshua Bloch
- “The Java Compiler” Tutorial by Oracle
- “In-Depth Guide to Java Compilation and Execution” Course on Udemy
Let me know if you would like me to expand or modify any part of this article draft. I can provide additional details wherever needed. Please feel free to give feedback so I can improve the content.
Try out our free resume checker service where our Industry Experts will help you by providing resume score based on the key criteria that recruiters and hiring managers are looking for.